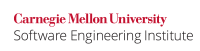
...
Code Block | ||
---|---|---|
| ||
class Test {
static long i = 0;
static void one() { i++; }
static void two() {
System.out.println("i =" + i);
}
}
|
then
...
method
...
two()
...
could
...
occasionally
...
...
a
...
value
...
for
...
i
...
that
...
is
...
not
...
one
...
more
...
than
...
the
...
previous
...
value
...
printed
...
by
...
two()
...
.
...
A
...
similar
...
problem
...
occurs
...
if
...
i
...
is
...
declared
...
as
...
a
...
double
.
Compliant Solution
This compliant solution declares the variables as volatile
. Writes and reads of volatile long and double values are always atomic.
Code Block | ||
---|---|---|
| ||
}}.
h2. Compliant Solution
This compliant solution declares the variables as {{volatile}}. Writes and reads of volatile long and double values are always atomic.
{code:bgColor=#FFcccc}
class Test {
static long i = 0;
static void one() { i++; }
static void two() {
System.out.println("i =" + i);
}
}
|
Compliant Solution
This compliant solution synchronizes calls to methods one()
and two()}}. This guarantees these two method calls are treated as atomic operation, including reading and writing to the variable i
. Consequently, there is no need to qualify i
as a volatile type.
Code Block | ||
---|---|---|
| ||
class Test {
static long i = 0;
static void synchronized one() { i++; }
static synchronized void two() {
System.out.println("i =" + i);
}
}
class Safe implements Runnable {
private long value1;
private double value2;
public synchronized void run() {
value1++;
value2++;
}
// ...
}
|
Consequently, there is no need to qualify i
as a volatile type.
Risk Assessment
Failure to ensure the atomicity of operations involving 64-bit values in multithreaded applications can result in reading and writing indeterminate values.
...