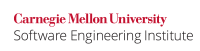
...
Notably, this applies only to fields and not to the contents of arrays or objects that are declared volatile
. A thread may not observe a recent write to the array's element or object's field from another thread.
Noncompliant Code Example (Properties
)
This noncompliant code example declares an instance field of type Properties
as volatile
. The field can be mutated using the put()
method.
...
Even if the client thread sees the new reference to properties
, the object state that it observes may change in the meantime. The Java Memory Model does not guarantee that the properties
field will have been properly initialized when it is necessary. Because the object is not immutable, it is unsafe for use in a multi-threaded environment.
Compliant Solution (immutable)
This compliant solution renders the Foo
class effectively immutable. Consequently, once it is properly constructed, no thread can modify properties
and cause a race condition.
...
The obvious drawback of this solution is that the put()
method cannot be accommodated if the goal is to ensure immutability. The Foo
and Properties
classes are both effectively immutable. They are not truly immutable because the Properties
class is not final.
Compliant Solution (the cheap read-write lock trick)
This compliant solution uses explicit synchronization to ensure thread safety. It declares the object volatile
to guard retrievals that use the getter method. A synchronized setter method is used to set the value of the Properties
object.
...
This compliant solution has the advantage that it can accommodate the setter method. Declaring the object as volatile
for safe publication using getter methods is cheaper in terms of performance, than declaring the getters as synchronized
. However, synchronizing the setter methods is mandatory.
Noncompliant Code Example (Arrays)
This noncompliant code example shows an array that is declared volatile
. It appears that, when a value is written by a thread to one of the array elements, it will be visible to other threads immediately. This is misleading because the volatile
keyword just makes the array reference visible to all threads and does not affect the actual data contained within the array.
...
It is possible for one thread to set a new value to arr1
while another thread attempts to read the value of arr1
, with the result that the reading thread receives an inconsistent value for arr1
.
Compliant Solution (AtomicIntegerArray
This compliant solution suggests using the java.util.concurrent.atomic.AtomicIntegerArray
concurrency utility. Using its set(index, value)
method ensures that the write is atomic and the resulting value is immediately visible to other threads. The other threads can retrieve a value from a specific index by using the get(index)
method.
Code Block | ||
---|---|---|
| ||
AtomicIntegerArray aia = new AtomicIntegerArray(5); // ... aia.set(1, 10); |
Risk Assessment
Failing to synchronize access to shared mutable data can cause different threads to observe different states of the object.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON11-J | medium | probable | medium | P8 | L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[Goetz 07|AA. Java References#Goetz 07]\] Pattern #2: "one-time safe publication" \[[JLS 05|AA. Java References#JLS 05]\] |
...