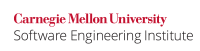
Declaring an object volatile
in order to ensure visibility of the most up-to-date object state does not work without the use of explicit synchronization, unless the object is effectively immutable.
Wiki Markup |
---|
In the absence of synchronization, the effect of declaring an object reference {{volatile}} is that when one thread sets the object to a new value, other threads can see the change immediately. In other words, the new value is immediately visible. If the object is immutable, then this has the effect that other threads see a clear change in the object's state. However, if one thread modifies an object, then other threads may see a partially-modified object, or an object in a (temporarily) inconsistent state \[[Goetz 07|AA. Java References#Goetz 07]\]. {{volatile}} does not prevent this. |
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 05|AA. Java References#JLS 05]\], section, 8.3.1.4 {{volatile}} Fields: |
...
Notably, this applies only to fields and not to the contents of arrays or objects that are declared volatile
. A thread may not observe a recent write to the array's element or object's field from another thread.
Noncompliant Code Example (Properties
)
This noncompliant code example declares an instance field of type Properties
as volatile
. The field can be mutated using the put()
method.
Code Block | ||
---|---|---|
| ||
public class Foo {
private volatile Properties properties;
public Foo() {
properties = new Properties();
// ... load some useful values into properties ...
}
public String get(String s) {
return properties.getProperty(s);
}
public void put(String key, String value) {
// Perform validation of value
properties.setProperty(key, value);
}
}
|
This class permits a race condition. If one thread calls get()
while another thread calls put()
, then the first thread might receive a stale value, or an internally inconsistent value from the properties
object. Being declared volatile
does not
Even if the client thread sees the new reference to properties
, the object state that it observes may change in the meantime. The Java Memory Model does not guarantee that the properties
field will have been properly initialized when it is necessary. Because the object is not immutable, it is unsafe for use in a multi-threaded environment.
Compliant Solution (immutable)
This compliant solution renders the Foo
class effectively immutable. Consequently, once it is properly constructed, no thread can modify properties
and cause a race condition.
Code Block | ||
---|---|---|
| ||
public class Foo {
private Properties properties;
public Foo() {
properties = new Properties();
// ... load some useful values into properties ...
}
public String get(String s) {
return properties.getProperty(s);
}
}
|
The obvious drawback of this solution is that the put()
method cannot be accommodated if the goal is to ensure immutability. The Foo
and Properties
classes are both effectively immutable. They are not truly immutable because the Properties
class is not final.
Compliant Solution (the cheap read-write lock trick)
This compliant solution uses explicit synchronization to ensure thread safety. It declares the object volatile
to guard retrievals that use the getter method. A synchronized setter method is used to set the value of the Properties
object.
Code Block | ||
---|---|---|
| ||
public class Foo {
private volatile Properties properties;
public Foo() {
properties = new Properties();
// ... load some useful values into properties ...
}
public String get(String s) {
return properties.getProperty(s);
}
public synchronized void put(String key, String value) {
// Perform validation of value
properties.setProperty(key, value);
}
}
|
This compliant solution has the advantage that it can accommodate the setter method. Declaring the object as volatile
for safe publication using getter methods is cheaper in terms of performance, than declaring the getters as synchronized
. However, synchronizing the setter methods is mandatory.
Noncompliant Code Example (Arrays)
This noncompliant code example shows an array that is declared volatile
. It appears that, when a value is written by a thread to one of the array elements, it will be visible to other threads immediately. This is misleading because the volatile
keyword just makes the array reference visible to all threads and does not affect the actual data contained within the array.
Code Block | ||
---|---|---|
| ||
class Unsafe { private volatile int[] arr = new int[20]; // ... } arr[21] = 10; |
It is possible for one thread to set a new value to arr1
while another thread attempts to read the value of arr1
, with the result that the reading thread receives an inconsistent value for arr1
.
Compliant Solution (AtomicIntegerArray
This compliant solution suggests using the java.util.concurrent.atomic.AtomicIntegerArray
concurrency utility. Using its set(index, value)
method ensures that the write is atomic and the resulting value is immediately visible to other threads. The other threads can retrieve a value from a specific index by using the get(index)
method.
Code Block | ||
---|---|---|
| ||
class Safe { AtomicIntegerArray aia = new AtomicIntegerArray(5); // Other code } ... aia.set(1, 10); |
Risk Assessment
The assumption that the contents of an array declared volatile
, are volatile
, can lead to stale readsFailing to synchronize access to shared mutable data can cause different threads to observe different states of the object.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON11-J | low medium | probable | medium | P4 P8 | L3 L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[Goetz 07|AA. Java References#Goetz 07]\] Pattern #2: "one-time safe publication"
\[[JLS 05|AA. Java References#JLS 05]\] |
...
CON10FIO36-J. Methods that override synchronized methods must also possess synchronization capabilities 11. Concurrency (CON) CON12-J. Avoid deadlock by requesting locks in the proper orderDo not create multiple buffered wrappers on an InputStream 09. Input Output (FIO) 09. Input Output (FIO)