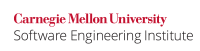
...
Code Block |
---|
public class Widget {
private int noOfComponents;
public Widget(int noOfComponents) {
this.noOfComponents = noOfComponents;
}
public int getNoOfComponents() {
return noOfComponents;
}
public void setNoOfComponents(int noOfComponents) {
this.noOfComponents = noOfComponents;
}
// Also overrides hashcode() (code is omitted) ...
public boolean equals(Object o) {
if (o == null || !(o instanceof Widget)) {
return false;
}
// check for negative components
if (noOfComponents < 0) {
return false;
}
Widget widget = (Widget) o;
return this.noOfComponents == ((Widget) o).getNoOfComponents();
}
}
public class Navigator extends Widget {
public Navigator(int noOfComponents) {
super(noOfComponents);
}
@Override
public boolean equals(Object o) {
if (o == null) {
return false;
}
return true;
}
}
public class LayoutManager {
private Set<Widget> layouts = new HashSet<Widget>();
public void addWidget(Widget widget) {
if (!layouts.contains(widget)) {
layouts.add(widget);
}
}
public int getLayoutSize() {
return layouts.size();
}
} |
...