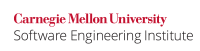
Null pointer dereferencing occurs when a variable bound to the null
variable value is treated as if it were a valid object reference and used without checking its state. This condition results in a NullPointerException
, and which can also in turn result in a denial of service. The denial of service is caused by the exception interrupting the program or thread, and probably causing it to terminate. Termination is likely because catching NullPointerExceptions
is generally discouraged (see ERR08-J. Do not catch NullPointerException or any of its ancestors).
Consequently, code must never dereference null pointers must never be dereferenced. That is, there must not exist some selection of inputs to a unit of code such that the code will dereference a null pointer. Note that the context in which code is defined can impact the determination of conformance to this rule, as shown in the second Noncompliant Code Example and (corresponding) second Compliant Solution.
Noncompliant Code Example
...
Code Block | ||
---|---|---|
| ||
public static int cardinality(Object obj, final Collection col) {
int count = 0;
if (col == null) {
return count;
}
Iterator it = col.iterator();
while (it.hasNext()) {
Object elt = it.next();
if ((null == obj && null == elt) ||
(null != obj && obj.equals(elt))) {
count++;
}
}
return count;
}
|
Explicit null checks as shown here an acceptable approach to eliminating null pointer dereferences.
Noncompliant Code Example
This noncompliant code example defines an isName()
method that takes a String argument and returns true if the given string is a valid name. A valid name is defined as two capitalized words separated by one or more spaces.
Code Block | ||
---|---|---|
| ||
boolean isName(String s) { String names[] = s.split(" "); if (names.length != 2) { return false; } return (isCapitalized(names[0]) && isCapitalized(names[1])); } |
Method isName
is not compliant with this rule because a null
argument will result in isName
dereferencing a null pointer.
Compliant Solution
This compliant solution demonstrates that the context in which code appears can impact its compliance. The example once again includes the isName
method, but this time as part of a more general method that tests string arguments.
Code Block | ||
---|---|---|
| ||
public class Foo {
private boolean isName(String s) {
String names[] = s.split(" ");
if (names.length != 2) {
return false;
}
return (isCapitalized(names[0]) && isCapitalized(names[1]));
}
public boolean testString(String s) {
if (s == null) return false;
else return isName(s);
}
}
|
In this example, we have made isName
a private method with only one caller in its containing class. The calling method, testString
, is written so as to guarantee that isName
is always called with a valid string reference. Therefore, the class as whole conforms with this rule, even though isName
in isolation does not. In general, inter-procedural reasoning of this sort is an acceptable approach to avoiding null pointer dereferences.
Exceptions
EXP01-EX0: A method may dereference an object-typed parameter without testing it for null guarantee that it is a valid object reference if the method documents that it (potentially) throws a NullPointerException
.EXP01-EX1: Private methods may dereference an object parameter without testing it for null. This is permitted because anyone with the ability to invoke the method can also access its source code to see that it might throw NullPointerException
, either via the throws
clause of in the method comments.
Risk Assessment
Dereferencing a null
pointer can lead to a denial of service. In multithreaded programs, null pointer dereferences can violate cache coherency policies and can cause resource leaks.
...
Wiki Markup |
---|
Java Web Start applications and applets particular to JDK version 1.6, prior to update 4, were affected by a bug that had some noteworthy security consequences. In some isolated cases, the application or applet's attempt to establish an HTTPS connection with a server generated a {{NullPointerException}} \[[SDN 2008|AA. References#SDN 08]\]. The resulting failure to establish a secure HTTPS connection with the server caused a denial of service. Clients were temporarily forced to use an insecure HTTP channel for data exchange. |
Related Guidelines
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="30abbdb85d04b5d3-599ff242-4c864213-8e87a15d-e0534f0eb0d04d20dae25ae4"><ac:plain-text-body><![CDATA[ | [ISO/IEC TR 24772:2010 | http://www.aitcnet.org/isai/] | Null Pointer Dereference [XYH] | ]]></ac:plain-text-body></ac:structured-macro> |
CWE-476. NULL pointer dereference |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a74b3b9ab7671fa3-779f2991-41f040a0-9b5c91ad-c0ed5883a35ad62e667548b2"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. References#API 06]] | [Method | http://java.sun.com/javase/6/docs/api/java/security/AccessController.html#doPrivileged(java.security.PrivilegedAction)] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="295f1077bad8d811-f6df97b6-438d4ffc-a9b4be80-a0fba4aed3fc15bfbdf8fd08"><ac:plain-text-body><![CDATA[ | [[Hovemeyer 2007 | AA. References#Hovemeyer 07]] |
| ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="9073e2a3721b07ae-b6999e85-4710497d-980799a8-4f3c97e3c6915babbe796b94"><ac:plain-text-body><![CDATA[ | [[Reasoning 2003 | AA. References#Reasoning 03]] | Defect ID 00-0001 | ]]></ac:plain-text-body></ac:structured-macro> | |
| Null Pointer Dereference | ||||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="c26a4274b6432cc1-f238e869-46844849-802d9c3e-ec2398b9fb2e28cc2d37437a"><ac:plain-text-body><![CDATA[ | [[SDN 2008 | AA. References#SDN 08]] | [Bug ID 6514454 | http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=6514454] | ]]></ac:plain-text-body></ac:structured-macro> |
...