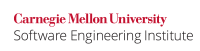
Wiki Markup |
---|
Many programs must address the problem of handling a series of incoming requests. One simple concurrency strategy is the Threadthread-Perper-Messagemessage design pattern, which uses a new thread for each request \[[Lea 2000|AA. Bibliography#Lea 00]\]. This pattern is generally preferred over sequential executions of time-consuming, I/O-bound, session-based, or isolated tasks. |
Wiki Markup |
---|
However, the pattern also introduces additional overheads not seen in sequential execution, including the time and resource required for thread-creation and scheduling, for task processing, for resource allocation and deallocation, and for frequent context switching \[[Lea 2000|AA. Bibliography#Lea 00]\]. Furthermore, an attacker can cause a denial of service by overwhelming the system with too many requests all at once, causing the system to become unresponsive rather than degrading gracefully. This can lead to a denial of service. From a safety perspective, one component can exhaust all resources duebecause toof an intermittent error, consequently starving all other components. |
...
This noncompliant code example demonstrates the Threadthread-Perper-Message message design pattern. The RequestHandler
class provides a public static factory method so that callers can obtain its instance. The handleRequest()
method is subsequently invoked to handle each request in its own thread.
Code Block | ||
---|---|---|
| ||
class Helper { public void handle(Socket socket) { //... } } final class RequestHandler { private final Helper helper = new Helper(); private final ServerSocket server; private RequestHandler(int port) throws IOException { server = new ServerSocket(port); } public static RequestHandler newInstance() throws IOException { return new RequestHandler(0); // Selects next available port } public void handleRequest() { new Thread(new Runnable() { public void run() { try { helper.handle(server.accept()); } catch (IOException e) { // Forward to handler } } }).start(); } } |
The Threadthread-Perper-Message message strategy fails to provide graceful degradation of service. As threads are created, processing continues normally until some scarce resource is exhausted. For example, a system may allow only a limited number of open file descriptors, even though additional threads can be created to serve requests. When the scarce resource is memory, the system may fail abruptly, resulting in a denial of service.
Compliant Solution (
...
Thread Pool)
Wiki Markup |
---|
This compliant solution uses a fixed thread pool that places a strict limit on the number of concurrently executing threads. Tasks submitted to the pool are stored in an internal queue. This prevents the system from being overwhelmed when attempting to respond to all incoming requests and allows it to degrade gracefully by serving a fixed maximum number of simultaneous clients \[[Tutorials 2008|AA. Bibliography#Tutorials 08]\]. |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
TPS00-J | low | probable | high | P2 | L3 |
Related Vulnerabilities
...
Related Guidelines
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a35c41f414ba8cc9-2b2088cd-40cb419d-96e89728-413b45d0af8bc5cc508c5451"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | [Interface Executor | http://java.sun.com/j2se/1.5.0/docs/api/java/util/concurrent/Executor.html] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="eec68b9c5d5a6476-83d2648b-45c349ca-ac1bb136-019da04c2fdc887179e393f6"><ac:plain-text-body><![CDATA[ | [[Lea 2000 | AA. Bibliography#Lea 00]] | Section 4.1.3 Thread-Per-Message and 4.1.4 Worker Threads | ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="6cd4b90e41546631-ff16d1b5-400e467b-a11884da-a239c5dfa319abfa7ea0f1e7"><ac:plain-text-body><![CDATA[ | [[Tutorials 2008 | AA. Bibliography#Tutorials 08]] | [Thread Pools | http://java.sun.com/docs/books/tutorial/essential/concurrency/pools.html] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="514eb5adc0660d1d-1ed85f1a-41cd4e08-a80faa17-36e86a36cb657272d77898bb"><ac:plain-text-body><![CDATA[ | [[Goetz 2006 | AA. Bibliography#Goetz 06]] | Chapter 8, Applying Thread Pools | ]]></ac:plain-text-body></ac:structured-macro> |
...