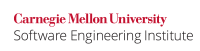
...
Code Block | ||||
---|---|---|---|---|
| ||||
import java.io.*; class OpenedFile implements Serializable { public String filename; public BufferedReader reader; public OpenedFile(String _filename) throws FileNotFoundException { filename = _filename; init(); } private void init() { throws FileNotFoundException try { reader = new BufferedReader(new FileReader(filename)); } catch (FileNotFoundException e) { } } private void writeObject(ObjectOutputStream out) throws IOException { out.writeUTF(filename); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { filename = in.readUTF(); init(); } } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
import java.io.*; interface Whitelist { public boolean has(String className); } class WhitelistedObjectInputStream extends ObjectInputStream { Whitelist whitelist; public WhitelistedObjectInputStream(InputStream inputStream) throws IOException { super(inputStream); } public void setWhitelist(Whitelist wl) { whitelist = wl; } } class OpenedFile implements Serializable { public String filename; public BufferedReader reader; public OpenedFile(String _filename) throws FileNotFoundException { filename = _filename; init(); } private void init() { trythrows FileNotFoundException { reader = new BufferedReader(new FileReader(filename)); } catch (FileNotFoundException e) { } } private void writeObject(ObjectOutputStream out) throws IOException { out.writeUTF(filename); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { boolean isWhitelisted = ((in instanceof WhitelistedObjectInputStream) && ((WhitelistedObjectInputStream) in).whitelist.has(this.getClass().getName())); if (!isWhitelisted) { throw new SecurityException("Attempted to deserialize unexpected class."); } filename = in.readUTF(); init(); } } |
...