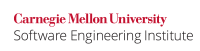
...
Use of the strictfp
modifier lacks has no impact on platforms that lack platform-specific floating point behavior. It can have substantial impact, however, on both the efficiency and the result values of floating point computations when executing on platforms that implement platform-specific floating point behavior. On these platforms, use of the strictfp
modifier increases the likelihood that intermediate operations will overflow or underflow because it restricts the representable range and precision of intermediate values; it can also reduce computational efficiency. These issues are unavoidable when portability is the main concern.
...
Usage | Strictness Behavior |
---|---|
Class | All code in the class including (instance, variable, static initializers), code in nested classes |
Method | All code within the method is subject to strictness constraints |
Interface | All code in the any class that implements the interface is also strict |
...
This noncompliant code example does not mandate strictfp
computation. Double.MAX_VALUE
is being multiplied by 1.1 and reduced back by dividing by 1.1, according to the evaluation order. If Double.MAX_VALUE
is indeed the maximum value permissible by the platform, the calculation will yield the result infinity
.
However, if the platform provides extended floating-point support, this program might print a numeric result roughly equivalent to Double.MAX_VALUE
.
JVM implementations are not required to report an overflow resulting from the initial multiplication, although they can choose to treat this case as strictfp
. The ability to use extended exponent ranges to represent intermediate values is implementation defined.
Code Block | ||
---|---|---|
| ||
class StrictfpExample { public static void main(String[] args) { double d = Double.MAX_VALUE; System.out.println("This value \"" + ((d * 1.1) / 1.1) + "\" cannot be represented as double."); } } |
Compliant Solution
To be compliantFor maximum portability, use the strictfp
modifier within an expression (class, method, or interface) to guarantee that intermediate results do not vary because of implementation-defined compiler optimizations or by design. This code snippet The calculation in this compliant solution is guaranteed to return positive INFINITY
produce infinity
because of the intermediate overflow condition, regardless of what floating-point support is provided by the platform.
Code Block | ||
---|---|---|
| ||
strictfp class StrictfpExample { public static void main(String[] args) { double d = Double.MAX_VALUE; System.out.println("This value \"" + ((d * 1.1) / 1.1) + "\" cannot be represented as double."); } } |
...
Failure to use the strictfp
modifier can result in platformimplementation-defined behavior, with respect to the accuracy of floating point operations.
...