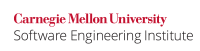
...
Code Block | ||
---|---|---|
| ||
float i = Float.MIN_VALUE; float j = Float.MAX_VALUE; if ((i < Short.MIN_VALUE) || (i > Short.MAX_VALUE) || (j < Short.MIN_VALUE) || (j > Short.MAX_VALUE)) { throw new ArithmeticException ("Value is out of range"); } short b = (short) i; short c = (short) j; //other operations |
Noncompliant Code Example (double
Conversion to float
)
The narrowing primitive conversions in this noncompliant code example suffer from loss in the magnitude of the numeric value, as well as a loss of precision. Becuase Double.MAX_VALUE
is larger than Float.MAX_VALUE
, c
receives the value infinity
. And because Double.MIN_VALUE
is smaller than Float.MIN_VALUE
, d
receives the value 0
.
Code Block | ||
---|---|---|
| ||
double i = Double.MIN_VALUE;
double j = Double.MAX_VALUE;
float b = (float) i;
float c = (float) j;
|
Compliant Solution (double
Conversion to float
)
Perform range checks on both i
and j
variables before proceeding with the conversions.
Code Block | ||
---|---|---|
| ||
double i = Double.MIN_VALUE;
double j = Double.MAX_VALUE;
if ((i < Float.MIN_VALUE) || (i > Float.MAX_VALUE) ||
(j < Float.MIN_VALUE) || (j > Float.MAX_VALUE)) {
throw new ArithmeticException ("Value is out of range");
}
float b = (float) i;
float c = (float) j;
//other operations
|
Exceptions
EXP13-EX1: Since Java's narrowing conventions are well-defined, they may be intentinally applied by programmers, and so their outputs would be expected and perfectly reasonable. Code that accounts for potential truncation, and documents this, is concisered a valid exception to this rule.
Risk Assessment
Casting a numeric value to a narrower type can result in information loss related to the sign and magnitude of the numeric value. Consequently, data can be misrepresented or interpreted incorrectly.
...