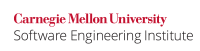
...
The strict behavior cannot be inherited by a subclass that extends a strictfp
superclass. An overriding method can independently choose to be strictfp
when the overridden method is not or vice versa.
Noncompliant Code Example
This noncompliant code example does not mandate strictfp
computation. Double.MAX_VALUE
is being multiplied by 1.1 and reduced back by dividing by 1.1, according to the evaluation order. If Double.MAX_VALUE
is indeed the maximum value permissible by the platform, the calculation will yield the result infinity
.
...
Code Block | ||
---|---|---|
| ||
class Example { public static void main(String[] args) { double d = Double.MAX_VALUE; System.out.println("This value \"" + ((d * 1.1) / 1.1) + "\" cannot be represented as double."); } } |
Compliant Solution
For maximum portability, use the strictfp
modifier within an expression (class, method, or interface) to guarantee that intermediate results do not vary because of implementation-defined compiler optimizations or by design. The calculation in this compliant solution is guaranteed to produce infinity
because of the intermediate overflow condition, regardless of what floating-point support is provided by the platform.
Code Block | ||
---|---|---|
| ||
strictfp class Example { public static void main(String[] args) { double d = Double.MAX_VALUE; System.out.println("This value \"" + ((d * 1.1) / 1.1) + "\" cannot be represented as double."); } } |
Risk Assessment
Failure to use the strictfp
modifier can result in implementation-defined behavior, with respect to the accuracy of floating point operations.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FLP04-J | low | unlikely | high | P1 | L3 |
Automated Detection
Sound automated detection of violations of this guideline are not feasible in the general case.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard FLP00-C. Understand the limitations of floating point numbers
Bibliography
Wiki Markup |
---|
\[[Darwin 2004|AA. Bibliography#Darwin 04]\] Ensuring the Accuracy of Floating-Point Numbers \[[JLS 2005|AA. Bibliography#JLS 05]\] [Section 15.4|http://java.sun.com/docs/books/jls/third_edition/html/expressions.html#15.4], "FP-strict Expressions" \[[JPL 2006|AA. Bibliography#JPL 06]\] 9.1.3. Strict and Non-Strict Floating-Point Arithmetic \[[McCluskey 2001|AA. Bibliography#McCluskey 01]\] Making Deep Copies of Objects, Using strictfp, and Optimizing String Performance |
...