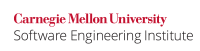
...
Code Block | ||
---|---|---|
| ||
public class InsecureServlet extends HttpServlet {
private UserDAO userDAO;
// ...
private String login(HttpServletRequest request) {
List<String> errors = new ArrayList<String>();
request.setAttribute("errors", errors);
String username = request.getParameter("username");
char[] password = request.getParameter("password").toCharArray();
// Basic input validation
if(!username.matches("[\\w]*") || !password.toString().matches("[\\w]*")) {
errors.add("Incorrect user name or password format.");
return "error.jsp";
}
UserBean dbUser = this.userDAO.lookup(username);
if(!dbUser.checkPassword(password)) {
errors.add("Passwords do not match.");
return "error.jsp";
}
HttpSession session = request.getSession();
// Invalidate old session id
session.invalidate();
// Generate new session id
session = request.getSession(true);
// Set session timeout to one hour
session.setMaxInactiveInterval(60*60);
// Store user bean within the session
session.setAttribute("user", dbUser.getUsername());
// Clear password char array
Arrays.fill(password, ' ');
return "welcome.jsp";
}
}
|
...