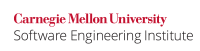
...
Avoid using home-brewed cryptographic algorithms; such algorithms will almost certainly introduce unnecessary vulnerabilities. Applications that apply home-brewed "cryptography" in the readObject()
and writeObject()
methods are prime examples of anti-patterns.
...
This noncompliant code example simply serializes then deserializes the map. Consequently, the map can be serialized and transferred across different business tiers. Unfortunately, there are no the example lacks any safeguards against byte stream manipulation attacks while the binary data is in transit. Likewise, anyone can reverse-engineer the serialized stream data to recover the data in the HashMap
.
Code Block | ||
---|---|---|
| ||
public static void main(String[] args) throws IOException, ClassNotFoundException { // Build map SerializableMap<String, Integer> map = buildMap(); // Serialize map ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data")); out.writeObject(map); out.close(); // Deserialize map ObjectInputStream in = new ObjectInputStream(new FileInputStream("data")); map = (SerializableMap<String, Integer>) in.readObject(); in.close(); // Inspect map InspectMap(map); } |
If the data in the map is were sensitive, this example violates would also violate rule SER03-J. Do not serialize unencrypted, sensitive data.
...
Code Block | ||
---|---|---|
| ||
public static void main(String[] args) throws IOException, GeneralSecurityException, ClassNotFoundException { // Build map SerializableMap<String, Integer> map = buildMap(); // Generate sealing key & seal map KeyGenerator generator; generator = KeyGenerator.getInstance("AES"); generator.init(new SecureRandom()); Key key = generator.generateKey(); Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, key); SealedObject sealedMap = new SealedObject(map, cipher); // Generate signing public/private key pair & sign map KeyPairGenerator kpg = KeyPairGenerator.getInstance("DSA"); KeyPair kp = kpg.generateKeyPair(); Signature sig = Signature.getInstance("SHA1withDSA"); SignedObject signedMap = new SignedObject(sealedMap, kp.getPrivate(), sig); // Serialize map ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data")); out.writeObject(signedMap); out.close(); // Deserialize map ObjectInputStream in = new ObjectInputStream(new FileInputStream("data")); signedMap = (SignedObject) in.readObject(); in.close(); // Unsign Verify signature and retrieve map if (!signedMap.verify(kp.getPublic(), sig)) { throw new GeneralSecurityException("Map failed verification"); } sealedMap = (SealedObject) signedMap.getObject(); // Unseal map cipher = Cipher.getInstance("AES"); cipher.init(Cipher.DECRYPT_MODE, key); map = (SerializableMap<String, Integer>) sealedMap.getObject(cipher); // Inspect map InspectMap(map); } |
...
Code Block | ||
---|---|---|
| ||
public static void main(String[] args) throws IOException, GeneralSecurityException, ClassNotFoundException { // Build map SerializableMap<String, Integer> map = buildMap(); // Generate signing public/private key pair & sign map KeyPairGenerator kpg = KeyPairGenerator.getInstance("DSA"); KeyPair kp = kpg.generateKeyPair(); Signature sig = Signature.getInstance("SHA1withDSA"); SignedObject signedMap = new SignedObject(map, kp.getPrivate(), sig); // Generate sealing key & seal map KeyGenerator generator; generator = KeyGenerator.getInstance("AES"); generator.init(new SecureRandom()); Key key = generator.generateKey(); Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, key); SealedObject sealedMap = new SealedObject(signedMap, cipher); // Serialize map ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data")); out.writeObject(sealedMap); out.close(); // Deserialize map ObjectInputStream in = new ObjectInputStream(new FileInputStream("data")); sealedMap = (SealedObject) in.readObject(); in.close(); // Unseal map cipher = Cipher.getInstance("AES"); cipher.init(Cipher.DECRYPT_MODE, key); signedMap = (SignedObject) sealedMap.getObject(cipher); // Unsign Verify signature and retrieve map if (!signedMap.verify(kp.getPublic(), sig)) { throw new GeneralSecurityException("Map failed verification"); } map = (SerializableMap<String, Integer>) signedMap.getObject(); // Inspect map InspectMap(map); } |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="33036c85de55672f-49aded2f-45a54ddf-9a50af87-b2886d92518727bafacc9ba9"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] |
| ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="2e7f5da0969034ba-550d5d75-46644a60-9b0db237-5a98e18fa3317a488c8eab62"><ac:plain-text-body><![CDATA[ | [[Gong 2003 | AA. Bibliography#Gong 03]] | 9.10, Sealing Objects | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="c4f8baca220a32c2-db005921-46c249b4-8a898e7b-548b3f007353d8f06d8cd93e"><ac:plain-text-body><![CDATA[ | [[Harold 1999 | AA. Bibliography#Harold 99]] | Chapter 11, Object serialization, sealed objects | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="6a67e852d1ef0f0d-5bac873f-4baa47a2-afdf8090-a10617cbd284f9483aff7adf"><ac:plain-text-body><![CDATA[ | [[Neward 2004 | AA. Bibliography#Neward 04]] | Item 64, Use | ]]></ac:plain-text-body></ac:structured-macro> |
| Item 65, Use | |||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="35cc6894d153b402-6709e632-429a4801-b867b42d-e4579b6277a70eb26e8ffd94"><ac:plain-text-body><![CDATA[ | [[Steel 2005 | AA. Bibliography#Steel 05]] | Chapter 10, Securing the Business Tier, Obfuscated Transfer Object | ]]></ac:plain-text-body></ac:structured-macro> |
...