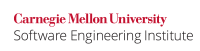
...
Because DateFormat
is not thread-safe [API 2011], the value for Date
returned by the parse()
method might fail to correspond to the str
argument:
...
This compliant solution creates and returns a new DateFormat
instance for each invocation of the parse()
method [API 2011]:
Code Block | ||
---|---|---|
| ||
final class DateHandler { public static java.util.Date parse(String str) throws ParseException { return DateFormat.getDateInstance(DateFormat.MEDIUM).parse(str); } } |
...
This compliant solution makes DateHandler
thread-safe by synchronizing statements within the parse()
method [API 2011]:
Code Block | ||
---|---|---|
| ||
final class DateHandler { private static DateFormat format = DateFormat.getDateInstance(DateFormat.MEDIUM); public static java.util.Date parse(String str) throws ParseException { synchronized (format) { return format.parse(str); } } } |
...
Technically, strict immutability of the referent is a stronger condition than is fundamentally required for safe publication. When it can be determined that a referent is thread-safe by design, the field that holds its reference may be declared volatile. However, this approach to using volatile
decreases maintainability and should be avoided.
Bibliography
...