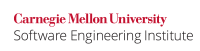
...
The non compliant example above can be resolved by using the HttpSesssion
class within the javax.servlet.http
package ?to store user information as opposed to cookies. Since HttpSession
objects are server-side, it is impossible for an attacker to gain access to the session information directly through cross-site scripting or man-in-the-middle attacks. Instead, a session id is stored within the cookie to refer to the user's HttpSession
object stored on the server. As a result, the attacker must first gain access to the session id and only then do they have a chance of gaining access to a user's account details.
Code Block | ||
---|---|---|
| ||
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.*;
import com.rhallsrv.insecure.model.UserDAO;
import com.rhallsrv.insecure.databeans.UserBean;
public class InsecureServlet extends HttpServlet {
private UserDAO userDAO;
// ...
private String login(HttpServletRequest request, HttpServletResponse response) {
List<String> errors = new ArrayList<String>();
request.setAttribute("errors", errors);
String username = request.getParameter("username");
String password = request.getParameter("password");
// Basic input validation
if(!username.matches("[\\w]*") || !password.matches("[\\w]*")) {
errors.add("Incorrect user name or password format.");
return "error.jsp";
}
UserBean dbUser = this.userDAO.lookup(username);
if(!dbUser.checkPassword(password)) {
errors.add("Passwords do not match.");
return "error.jsp";
}
HttpSession session = request.getSession();
session.invalidate(); // Invalidate old session id
session = request.getSession(true); // Generate new session id
session.setMaxInactiveInterval(2*60*60); // Set session timeout to two hours
session.setAttribute("user", dbUser); // Store user bean within the session
return "welcome.jsp";
}
}
|
...