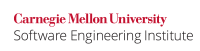
Integer variables are frequently intended to represent either a numeric value or a bitmap. Numeric values should be exclusively operated upon using arithmetic operations, while bitmaps should be exclusively operated upon using logical operations. However, it is frequently difficult for an analyzer to determine if the intended usage of a particular integer variable.
Performing Avoid performing bitwise and arithmetic operations on the same data does suggest confusion about the data . In particularstored in the variable. Unfortunately, bitwise operations are frequently performed on arithmetic values as a form of premature optimization. Bitwise operators include the unary operator ~ and the binary operators <<
, >>
, &
, ^, and |
. Although such operations are valid and will compile, they can reduce code readability. Declaring a variable as containing a numeric value or a bitmap makes the programmer's intentions clearer and the code more maintainable.
Left- and right-shift operators are often employed to multiply or divide a number by a power of 2. This compromises code readability and portability for the sake of often-illusory speed gains. The JVM will usually make such optimizations automatically, and unlike a programmer, the JVM can optimize for the implementation details of the current platform. This is also problematic in that right shifting is not equivalent to division for negative dividends.
Noncompliant Code Example (Left Shift)
...
Code Block | ||
---|---|---|
| ||
int x = -50; x = x + 2; x >>= 2; |
Although this code will perform the division correctlyUsing logical right shift for division produces an incorrect result when the dividend (x
in this example) contains a negative value. Although left shift can replace division for non-negative dividends, it is less readable and maintainable than actually making the division explicit.
...