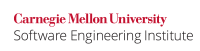
...
Code Block | ||
---|---|---|
| ||
class Example { double d = 0.0; public static void mainexample(String[] args) { float f = Float.MAX_VALUE; float g = Float.MAX_VALUE; double this.d = f * g; System.out.println("d (" + this.d ") might not be equal to " + (f * g)); } public static void main(String[] args) { Example ex = new Example(); ex.example(); } } |
The lost precision or magnitude would also have been lost if the value were stored to memory, for example to a field of type float
.
Compliant Solution
Code Block | ||
---|---|---|
| ||
strictfp class Example {
double d = 0.0;
public void example() {
float f = Float.MAX_VALUE;
float g = Float.MAX_VALUE;
this.d = f * g;
System.out.println("d (" + this.d ") might not be equal to " + (f * g));
}
public static void main(String[] args) {
Example ex = new Example();
ex.example();
}
}
|
Risk Assessment
Failure to use the strictfp
modifier can result in implementation-defined behavior, with respect to the accuracy of floating point operations.
...