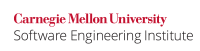
...
The minimum and maximum float
values are converted to minimum and maximum int
values (0x80000000
and 0x7fffffff
) respectively. The resulting short
values are the lower 16 bits of these values (0x0000
and 0xffff
. The resulting final values (0 and -1) might be unexpected.
Compliant Solution (Floating-point
...
to Integer Conversion)
This compliant solution range checks both the i
and j
variables before converting to the resulting integer type.
Code Block | ||
---|---|---|
| ||
float i = Float.MIN_VALUE; float j = Float.MAX_VALUE; if ((i < Short.MIN_VALUE) || (i > Short.MAX_VALUE) || (j < Short.MIN_VALUE) || (j > Short.MAX_VALUE)) { throw new ArithmeticException ("Value is out of range"); } short b = (short) i; short c = (short) j; //other operations |
Noncompliant Code Example (double
...
to float
Conversion)
The narrowing primitive conversions in this noncompliant code example suffer from a loss in the magnitude of the numeric value, as well as a loss of precision. Because Double.MAX_VALUE
is larger than Float.MAX_VALUE
, c
receives the value infinity
. And and because Double.MIN_VALUE
is smaller than Float.MIN_VALUE
, d
receives the value 0
.
Code Block | ||
---|---|---|
| ||
double i = Double.MIN_VALUE; double j = Double.MAX_VALUE; float b = (float) i; float c = (float) j; |
Compliant Solution (double
...
to float
Conversion)
Perform range checks on both i
and j
variables before proceeding with the conversions.
Code Block | ||
---|---|---|
| ||
double i = Double.MIN_VALUE; double j = Double.MAX_VALUE; if ((i < Float.MIN_VALUE) || (i > Float.MAX_VALUE) || (j < Float.MIN_VALUE) || (j > Float.MAX_VALUE)) { throw new ArithmeticException ("Value is out of range"); } float b = (float) i; float c = (float) j; //other operations |
Exceptions
EXP13NUM??-EX0: Java's narrowing conversions are both well-defined and portable; knowledgeable programmers can intentionally apply such conversions in contexts where their output is both expected and reasonable. Consequently, narrowing conversions are permitted when the code contains comments that document both the use of narrowing conversions and that the potential for truncation has been anticipated. A suitable comment might read: "// Deliberate narrowing cast of i; possible truncation OK"
...