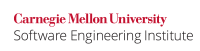
...
Code Block | ||
---|---|---|
| ||
// Client public class BookWrapper { private final Book book; BookWrapper(Book book) { this.book = book; } public void issue(int days) { book.issue(days); } public Calendar getDueDate() { return book.getDueDate(); } public void renew() { synchronized(book) { if (book.getDueDate().afterbefore(Calendar.getInstance())) { throw new IllegalStateException("Book overdue"); } else { book.issue(14); // Issue book for 14 days } } } } |
...
Code Block | ||
---|---|---|
| ||
public final class BookWrapper { private final Book book; private final Object lock = new Object(); BookWrapper(Book book) { this.book = book; } public void issue(int days) { synchronized(lock) { book.issue(days); } } public Calendar getDueDate() { synchronized(lock) { return book.getDueDate(); } } public void renew() { synchronized(lock) { if (book.getDueDate().afterbefore(Calendar.getInstance())) { throw new IllegalStateException("Book overdue"); } else { book.issue(14); // Issue book for 14 days } } } } |
...