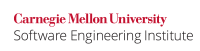
...
Method isProperName
()
is noncompliant because it may be called with a null
argument, resulting in a null pointer dereference.
Compliant Solution (Wrapped Method)
This compliant solution includes the same isProperName()
method implementation as the previous noncompliant example, but it is now a private method with only one caller in its containing class.
...
The calling method, testString()
, guarantees that isProperName
()
is always called with a valid string reference. As a result, the class conforms with this rule, even though a public isProperName()
method would not. Guarantees of this sort can be used to eliminate null pointer dereferences.
Compliant Solution (Optional Type)
This compliant solution uses an Optional String
instead of a String
object that may be null. The Optional
class ([API 2014] java.util.Optional
) was introduced in Java 8 to make dealing with possibly null objects easier, see [Urma 2014].
Code Block | ||
---|---|---|
| ||
public boolean isProperName(Optional<String> os) {
if (os.isPresent()) {
String names[] = os.get().split(" ");
if (names.length != 2) {
return false;
}
return (isCapitalized(names[0]) && isCapitalized(names[1]));
else {
return false;
}
}
|
The Optional class contains methods that can be used in the functional style of Java 8 to make programs shorter and more intuitive, as illustrated in the technical note by Urma cited above.
Exceptions
EXP01-EX0: A method may dereference an object-typed parameter without guarantee that it is a valid object reference provided that the method documents that it (potentially) throws a NullPointerException
, either via the throws
clause of the method or in the method comments. However, this exception should be relied upon sparingly.
...
[API 2006] | |
[API 2014] | Class java.util.Optional |
| |
Defect ID 00-0001 | |
| Null Pointer Dereference |
[SDN 2008] | |
[Urma 2014] | Tired of Null Pointer Exceptions? Consider Using Java SE 8's Optional! |
...