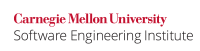
...
This noncompliant code example corrupts the data when string
contains characters that are not representable in the specified charset
.
Code Block | ||
---|---|---|
| ||
// Corrupts data on errors
public static byte[] toCodePage(String charset, String string)
throws UnsupportedEncodingException {
return string.getBytes(charset);
}
// Fails to detect corrupt data
public static String fromCodePage(String charset, byte[] bytes)
throws UnsupportedEncodingException {
return new String(bytes, charset);
}
|
...
Compliant Solution
The java.nio.charset.CharsetEncoder
class can transform a sequence of 16-bit Unicode characters into a sequence of bytes in a specific charset
, while the java.nio.charset.CharacterDecoder
class can reverse the procedure [API 2006].
...
Code Block | ||||
---|---|---|---|---|
| ||||
public static byte[] toCodePage(String charset, String string) throws IOException { Charset cs = Charset.forName(charset); CharsetEncoder coder = cs.newEncoder(); ByteBuffer bytebuf = coder.encode(CharBuffer.wrap(string)); byte[] bytes = new byte[bytebuf.limit()]; bytebuf.get(bytes); return bytes; } public static String fromCodePage(String charset,byte[] bytes) throws CharacterCodingException { Charset cs = Charset.forName(charset); CharsetDecoder coder = cs.newDecoder(); CharBuffer charbuf = coder.decode(ByteBuffer.wrap(bytes)); return charbuf.toString(); } |
Noncompliant Code Example
...