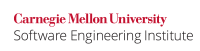
Java uses security managers and security policies together to prevent untrusted code from performing privileged operations. In standard installations, a restrictive system-wide systemwide security policy prevents instantiation of sensitive classes such as java.lang.ClassLoader
in the context of a web browser, for example. It remains critically important to ensure that untrusted code cannot indirectly use the privileges of trusted code to perform privileged operations.
Most APIs install security manager checks to prevent such privilege escalation attacks; some APIs fail to do so. These APIs are tabulated below. Note, however, that the loadLibrary
and load
APIs throw a security exception when the caller lacks permission to dynamically link the library code. These APIs are nevertheless listed as unsafe because they use the immediate caller's class loader to find and load the requested library. Moreover, because the loadLibrary
and load
APIs are typically used from within a doPrivileged
block defined in trusted code, untrusted callers can directly invoke it , without requiring any special permissions.
...
Security vulnerabilities can also arise even when untrusted code has been loaded by a class loader instance that is distinct from the class loader used to load the trusted code. When the untrusted code's class loader delegates to the trusted code's class loader, the untrusted code has visibility to the trusted code according to the declared visibility of the trusted code. In the absence of such a delegation relationship, the class loaders would ensure namespace separation; consequently, the untrusted code would be unable to observe members or to invoke methods belonging to the trusted code.
Consider, for example, untrusted code that is attempting to load a privileged class. Its class loader is permitted to delegate the class loading to the trusted class's class loader. This can result in privilege escalation, because the untrusted code's class loader may lack permission to load the requested privileged class. Further, if the trusted code accepts tainted inputs, the trusted code's class loader could load additional privileged — or even malicious — classes on behalf of the untrusted code.
...
In this noncompliant code example a call to System.loadLibrary()
is embedded in a doPrivileged
block. An unprivileged caller can maliciously invoke this piece of code using the same technique as above , because the doPrivileged
block allows security manager checks to be forgone for other callers on the execution chain.
Code Block | ||
---|---|---|
| ||
public void load(String libName) { AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary(libName); return null; } }); } |
Non-native Nonnative library code can also be susceptible to related security flaws. Loading a non-native nonnative safe library , may not directly expose a vulnerability; , but after loading an additional unsafe library, an attacker can easily exploit the safe library if it contains other vulnerabilities. Moreover, non-native nonnative libraries often use doPrivileged
blocks, making them lucrative targets.
...
This compliant solution reduces the accessibility of method load()
from public
to private
. Consequently, untrusted callers are prohibited from loading the awt
library. Also, the name of the library is hard-coded to reject the possibility of tainted values.
...
Limit the visibility of the method that uses this API. Do not operate on tainted inputs. This compliant solution hardcodes hard-codes the class's name.
Code Block | ||
---|---|---|
| ||
Class c = Class.forName("Foo"); // Explicitly hardcode |
...
This noncompliant code example returns an instance of java.sql.Connection
from trusted to untrusted code. Untrusted code that lacks the permissions required to create an a SQL connection can bypass these restrictions by using the acquired instance directly.
...
Ensure that instances of objects created using the unsafe methods are not returned to untrusted code. Furthermore, it It is preferable to reduce the accessibility of methods that perform sensitive operations and define wrapper methods that are accessible from untrusted code.
...