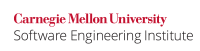
...
Code Block | ||
---|---|---|
| ||
class ReadNames { // ...other methods and variables public static final int fileSizeLimit = 1000000; public ReadNames(String filename) throws IOException { long size = Files.size( Paths.get( filename)); if (size > fileSizeLimit) { throw new IOException("File too large"); } else if (size == 0L) { throw new IOException("File size cannot be determined, possibly too large"); } this.input = new FileReader(filename); this.reader = new BufferedReader(input); } // ...other methods } |
Compliant Solution (Limited Length Input)
...
Code Block | ||
---|---|---|
| ||
class ReadNames { // ... other methods and variables public static String readLimitedLine(Reader reader, int limit) throws IOException { StringBuilder sb = new StringBuilder(); for (int i = 0; i < limit; i++) { int c = reader.read(); if (c == -1) { return null; } if (((char) c == '\n') || ((char) c == '\r')) { break; } sb.append((char) c); } return sb.toString(); } public static final int lineLengthLimit = 1024; public static final int lineCountLimit = 1000000; public void addNames() throws IOException { try { String newName; for (int i = 0; i < lineCountLimit; i++) { newName = readLimitedLine(reader, lineLengthLimit); if (newName == null || newName.equalsIgnoreCase("quit")) { break; } names.addElement(newName); System.out.println("adding " + newName); } } finally { input.close(); } } } |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="44d0143c8e4ab1ce-288286b2-4c5e463d-892baada-772f755ff10b600842400583"><ac:plain-text-body><![CDATA[ | [ISO/IEC TR 24772:2010 | http://www.aitcnet.org/isai/] | Resource Exhaustion [XZP] | ]]></ac:plain-text-body></ac:structured-macro> |
CWE-400. Uncontrolled resource consumption ("resource exhaustion") | ||||
| CWE-770. Allocation of resources without limits or throttling |
...
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="1a976190613f63c3-9e5561e2-440544a9-a7838696-157e9834fabb202e656bd693"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. References#API 06]] | Class | ]]></ac:plain-text-body></ac:structured-macro> | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="6c151fa2743b9096-bbff95c4-4f324766-bceda094-2eff26cca992d2975b089b59"><ac:plain-text-body><![CDATA[ | [[Java 2006 | AA. References#Java 06]] | [java – The Java application launcher | http://java.sun.com/javase/6/docs/technotes/tools/windows/java.html], Syntax for increasing the heap size | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="0315d40237fe701e-16c08e25-4e434403-93c4953c-8dd124a779f27024c5449bf4"><ac:plain-text-body><![CDATA[ | [[SDN 2008 | AA. References#SDN 08]] | [Serialization FAQ | http://java.sun.com/javase/technologies/core/basic/serializationFAQ.jsp] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="cb173627a1285f11-7dbaa89b-4cf542c9-95f6b4a3-0ffc8501f342fd26fea47522"><ac:plain-text-body><![CDATA[ | [[Sun 2003 | AA. References#Sun 03]] | Chapter 5, Tuning the Java Runtime System, [Tuning the Java Heap | http://docs.sun.com/source/817-2180-10/pt_chap5.html#wp57027] | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="4615e6c257c74098-3a293e58-4a924bc1-a16dac68-d2c2d96de4348e49ce664107"><ac:plain-text-body><![CDATA[ | [[Sun 2006 | AA. References#Sun 06]] | [Garbage Collection Ergonomics | http://java.sun.com/javase/6/docs/technotes/guides/vm/gc-ergonomics.html ], Default values for the Initial and Maximum Heap Size | ]]></ac:plain-text-body></ac:structured-macro> |
...