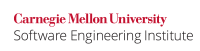
...
Code Block | ||
---|---|---|
| ||
public final class CreditCard implements Serializable {
// Private internal state
private String credit_card;
private static final String DEFAULT = "DEFAULT";
void performSecurityManagerCheck() throws AccessDeniedException {
// ...
}
void validateInput(String newCC) throws InvalidInputException {
// ...
}
public CreditCard() {
performSecurityManagerCheck();
// Initialize credit_card to default value
credit_card = DEFAULT;
}
// Allows callers to retrieve internal state
String getValue() {
performSecurityManagerCheck();
return credit_card;
}
// Allows callers to modify (private) internal state
public void changeCC(String newCC) {
if (credit_card.equals(newCC)) {
// No change
return;
} else {
performSecurityManagerCheck();
validateInput(newCC);
credit_card = newCC;
}
}
// writeObject() correctly enforces checks during serialization
private void writeObject(ObjectOutputStream out) throws IOException {
out.writeObject(credit_card);
}
// readObject() correctly enforces checks during deserialization
private void readObject(ObjectInputStream in) throws IOException {
in.defaultReadObject();
// If the deserialized name does not match the default value normally
// created at construction time, duplicate the checks
if (!DEFAULT.equals(credit_card)) {
validateInput(credit_card);
}
}
}
|
...
Code Block | ||
---|---|---|
| ||
public final class CreditCard implements Serializable { // ... all methods the same except the following: // Allows callers to retrieve internal state public String getValue() { // Check permission to get value performSecurityManagerCheck(); return somePublicValue; } //allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // No change return; } else { // Check permissions to modify credit_card performSecurityManagerCheck(); validateInput(newCC); credit_card = newCC; } } // writeObject() correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) throws IOException { // Duplicate check from getValue() performSecurityManagerCheck(); out.writeObject(credit_card); } // readObject() correctly enforces checks during deserialization private void readObject(ObjectInputStream in) throws IOException { in.defaultReadObject(); // If the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { performSecurityManagerCheck(); validateInput(credit_card); } } } |
...