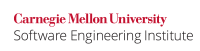
...
Code Block | ||
---|---|---|
| ||
public final class CreditCard implements Serializable { // Private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; void performSecurityManagerCheck() throws AccessDeniedException { // ... } void validateInput(String newCC) throws InvalidInputException { // ... } public CreditCard() throws AccessDeniedException { performSecurityManagerCheck(); // Initialize credit_card to default value credit_card = DEFAULT; } // Allows callers to modify (private) internal state public void changeCC(String newCC) throws InvalidInputException { if (credit_card.equals(newCC)) { // No change return; } else { performSecurityManagerCheck(); validateInput(newCC); credit_card = newCC; } } // readObject() correctly enforces checks during deserialization private void readObject(ObjectInputStream in) throws IOException, InvalidInputException { in.defaultReadObject(); // If the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { validateInput(credit_card); } } // Allows callers to retrieve internal state String getValue() { return credit_card; } // writeObject() correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) throws IOException { out.writeObject(credit_card); } } |
...
Code Block | ||
---|---|---|
| ||
public final class SecureCreditCard implements Serializable { // Private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; void performSecurityManagerCheck() throws AccessDeniedException { // ... } void validateInput(String newCC) throws InvalidInputException { // ... } public SecureCreditCard() throws AccessDeniedException { performSecurityManagerCheck(); // Initialize credit_card to default value credit_card = DEFAULT; } //allows callers to modify (private) internal state public void changeCC(String newCC) throws AccessDeniedException, InvalidInputException { if (credit_card.equals(newCC)) { // No change return; } else { // Check permissions to modify credit_card performSecurityManagerCheck(); validateInput(newCC); credit_card = newCC; } } // readObject() correctly enforces checks during deserialization private void readObject(ObjectInputStream in) throws IOException { in.defaultReadObject(); // If the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { performSecurityManagerCheck(); validateInput(credit_card); } } // Allows callers to retrieve internal state public String getValue() { // Check permission to get value performSecurityManagerCheck(); return somePublicValue; } // writeObject() correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) throws IOException { // Duplicate check from getValue() performSecurityManagerCheck(); out.writeObject(credit_card); } } |
...