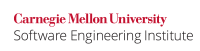
Do not operate on unvalidated or untrusted data (also known as tainted data) in a doPrivileged()
block. An attacker can supply malicious input that could result in privilege escalation attacks. Appropriate mitigations include hardcoding hard coding values rather than accepting arguments (when appropriate), or validating (a.k.a. sanitizing) data before the privileged operations.
...
Code Block | ||
---|---|---|
| ||
private void privilegedMethod(final String filename) throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(filename); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
Compliant Solution (
...
Input Validation)
This compliant solution explicitly hardcodes the name of the file and confines the variables used in the privileged block to the same method. This ensures that no malicious file can be loaded by exploiting the privileges of the corresponding codeinvokes a sanitization method (cleanAFilenameAndPath
) that can distinguish acceptable inputs from malicious inputs. Successful operation of the sanitization method indicates that the input is acceptable, and the doPrivileged
block can be executed.
Code Block | ||
---|---|---|
| ||
static private void privilegedMethod(final String filename) throws FileNotFoundException { final String FILEPATH cleanFilename; try { cleanFilename = "/path/to/protected/file/fn.ext"; private void privilegedMethod() throws FileNotFoundException { cleanAFilenameAndPath(filename); } catch (/* exception as per spec of cleanAFileNameAndPath */) { // log or forward to handler as appropriate based on specification // of cleanAFilenameAndPath } try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(FILEPATHcleanFilename); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
One potential drawback of this approach is that effective sanitization methods can be difficult to write. A benefit of this approach is that it works well in combination with taint analysis (see Risk Assessment).
Compliant Solution (
...
Built-in File Name and Path)
Sanitization of tainted inputs always carries the risk that the data is not fully sanitized. File and path name equivalence vulnerabilities and directory traversal vulnerabilities are both common examples of vulnerabilities arising from the improper sanitization path and file name inputs (see FIO04-J. Canonicalize path names before validating). A design that requires an unprivileged user to access an arbitrary, protected file (or other resource) is always suspect. Instead, you may want to consider using a hard code resource name or only allow the user to select from a list of options that are indirectly mapped to the resource names.
This compliant solution explicitly hard codes the name of the file and confines the variables used in the privileged block to the same method. This ensures that no malicious file can be loaded by exploiting the privileges of the corresponding codeThis compliant solution invokes a sanitization method (cleanAFilenameAndPath
) that can distinguish acceptable inputs from malicious inputs. Successful operation of the sanitization method indicates that the input is acceptable, and the doPrivileged
block can be executed.
Code Block | ||
---|---|---|
| ||
privatestatic void privilegedMethod(final String filename)FILEPATH throws FileNotFoundException { final String cleanFilename; try { cleanFilename = cleanAFilenameAndPath(filename); } catch (/* exception as per spec of cleanAFileNameAndPath */) { // log or forward to handler as appropriate based on specification // of cleanAFilenameAndPath } = "/path/to/protected/file/fn.ext"; private void privilegedMethod() throws FileNotFoundException { try { FileInputStream fis = (FileInputStream) AccessController.doPrivileged( new PrivilegedExceptionAction() { public FileInputStream run() throws FileNotFoundException { return new FileInputStream(cleanFilenameFILEPATH); } } ); // do something with the file and then close it } catch (PrivilegedActionException e) { // forward to handler and log } } |
...
Risk Assessment
Allowing tainted inputs in privileged operations can lead to privilege escalation attacks.
...