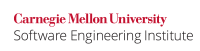
...
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200,5); division(200,0); // Divide by zero } catch (ArithmeticException ae) { throw new DivideByZeroException(); // DivideByZeroException extends Exception so is checked } catch (IOException ie) { ExceptionReporter.report(ex); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("I/O Exception occurred :" + ie.getMessage());Average: "+ average); } } |
Note that DivideByZeroException
is a custom exception type that extends Exception
.
The ExceptionReporter
class is documented in ERR00-J. Do not suppress or ignore checked exceptions.
Compliant Solution (Java 1.7)
Java 1.7 allows a single catch block to catch multiple exceptions. This allows one catch block to handle exceptions of different types, which prevents redundant code. This compliant solution catches the specific anticipated exceptions (ArithmeticException
and IOException
), and handles them with one catch clause. All other exceptions are permitted to propagate up the call stack.
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200,5); division(200,0); // Divide by zero } catch (ArithmeticException|IOException ex) { ExceptionReporter.report(ex); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("Average: "+ average); } } |
...
Wiki Markup |
---|
\[[Doshi 2003|AA. Bibliography#Doshi 03]\]
\[[JLS 2005|AA. Bibliography#JLS 05]\] [Chapter 11, Exceptions|http://java.sun.com/docs/books/jls/third_edition/html/exceptions.html]
\[[J2SE 2011|AA. Bibliography#J2SE 11]\] Catching Multiple Exception Types and Rethrowing Exceptions with Improved Type Checking
\[[MITRE 2009|AA. Bibliography#MITRE 09]\] [CWE ID 396|http://cwe.mitre.org/data/definitions/396.html] "Declaration of Catch for Generic Exception", [CWE ID 7|http://cwe.mitre.org/data/definitions/7.html] "J2EE Misconfiguration: Missing Error Handling", [CWE ID 537|http://cwe.mitre.org/data/definitions/537.html] "Information Leak Through Java Runtime Error Message", [CWE ID 536|http://cwe.mitre.org/data/definitions/536.html] "Information Leak Through Servlet Runtime Error Message"
\[[Muller 2002|AA. Bibliography#Muller 02]\]
\[[Rogue 2000|AA. Bibliography#Rogue 2000]\] Rule 87: Do not silently absorb a run-time or error exception
\[[Schweisguth 2003|AA. Bibliography#Schweisguth 03]\]
\[[Tutorials 2008|AA. Bibliography#tutorials 08]\] Exceptions|http://java.sun.com/docs/books/tutorial/essential/exceptions/index.html] |
...