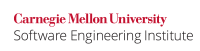
...
This noncompliant code example corrupts the data when string
contains characters that are not representable in the specified charset
.
Code Block | ||||
---|---|---|---|---|
| ||||
// Corrupts data on errors public static byte[] toCodePage(String charset, String string) throws UnsupportedEncodingException { return string.getBytes(charset); } // Fails to detect corrupt data public static String fromCodePage(String charset, byte[] bytes) throws UnsupportedEncodingException { return new String(bytes, charset); } |
...
This noncompliant code example attempts to append a string to a text file in the specified encoding. This is erroneous because the String
may contain unrepresentable characters.
Code Block | ||||
---|---|---|---|---|
| ||||
// Corrupts data on errors public static void toFile(String charset, String filename, String string) throws IOException { FileOutputStream stream = new FileOutputStream(filename, true); OutputStreamWriter writer = new OutputStreamWriter(stream, charset); writer.write(string, 0, string.length()); writer.close(); } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
public static void toFile(String filename, String string, String charset) throws IOException { Charset cs = Charset.forName(charset); CharsetEncoder coder = cs.newEncoder(); FileOutputStream stream = new FileOutputStream(filename, true); OutputStreamWriter writer = new OutputStreamWriter(stream, coder); writer.write(string, 0, string.length()); writer.close(); } |
Use the FileInputStream
and InputStreamReader
objects to read back the data from the file. InputStreamReader
accepts a optional CharsetDecoder
argument, which must be the same as that previously used for writing to the file.
...