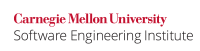
...
Avoid using home-brewed cryptographic algorithms; such algorithms almost certainly introduce unnecessary vulnerabilities. Applications that apply home-brewed "cryptography" in the readObject()
and writeObject()
methods are prime examples of anti-patterns.
Noncompliant Code Example
This noncompliant code example is capable of being serialized and transferred across different business tiers. Unfortunately, there are no safeguards against byte stream manipulation attacks while the binary data is in transit. Likewise, anyone can reverse engineer the stream data from its hexadecimal notation to reveal the data in the HashMap
.
Wiki Markup |
---|
Furthermore, Abadi and Needham have suggested \[[Abadi 1996|AA. Bibliography#Abadi 96]\] a useful principle of secure software design |
When a principal signs material that has already been encrypted, it should not be inferred that the principal knows the content of the message. On the other hand, it is proper to infer that the principal that signs a message and then encrypts it for privacy knows the content of the message.
Wiki Markup |
---|
The rationale is that any malicious party can intercept the originally signed encrypted message from the originator, strip the signature and add its own signature to the encrypted message. Both the malicious party, and the receiver have no information about the contents of the original message as it is encrypted and then signed (it can only be decrypted after verifying the signature). The receiver has no way of confirming the sender's identity unless the legitimate sender's public key is obtained over a secure channel. One of the three CCITT X.509 standard protocols was susceptible to such an attack \[[CCITT 1988|AA. Bibliography#CCITT 88]\]. |
The subsequent code examples all involve the following code sample. This code sample posits a map that is serializable, as well as a function to populate the map with interesting values, and a function to check the map for those values.
Code Block |
---|
Code Block | bgColor | #FFcccc
class SerializableMap<K,V> implements Serializable { final static long serialVersionUID = -2648720192864531932L; private HashMap<KMap<K,V> map; public SerializableMap() { map = new HashMap<K,V>(); } public Object getData(K key) { return map.get(key); } public void setData(K key, V data) { map.put(key, data); } } public class MapSerializer { static public SerializableMap< String, Integer> buildMap() { SerializableMap< String, Integer> map = new SerializableMap< String, Integer>(); map.setData("John Doe", new Integer( 123456789)); map.setData("Richard Roe", new Integer( 246813579)); return map; } static public void InspectMap(SerializableMap< String, Integer> map) { System.out.println("John Doe's number is " + map.getData("John Doe")); System.out.println("Richard Roe's number is " + map.getData("Richard Roe")); } public static void main(String[] args) { // ... } } |
Noncompliant Code Example
This noncompliant code example simply serializes the map and then deserializes it. Thus, the map is capable of being serialized and transferred across different business tiers. Unfortunately, there are no safeguards against byte stream manipulation attacks while the binary data is in transit. Likewise, anyone can reverse engineer the serialized stream data from its hexadecimal notation to reveal the data in the HashMap
.
Code Block | ||
---|---|---|
| ||
public static void main(String[] args)
throws IOException, ClassNotFoundException {
// Build map
SerializableMap< String, Integer> map = buildMap();
// Serialize map
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data"));
out.writeObject( map);
out.close();
// Deserialize map
ObjectInputStream in = new ObjectInputStream(new FileInputStream("data"));
map = (SerializableMap< String, Integer>) in.readObject();
in.close();
// Inspect map
InspectMap( map);
}
|
If the data in the map is considered sensitive, this example will also violate SER03-J. Do not serialize unencrypted, sensitive data.
...
Noncompliant Code Example (Seal)
To provide message confidentiality, use the javax.crypto.SealedObject
class. This class encapsulates a serialized object and encrypts (or seals) it. A strong cryptographic algorithm that uses a secure cryptographic key and padding scheme must be employed to initialize the Cipher
object parameter. The seal
and unseal
utility methods provide the encryption and decryption facilities respectively.
This noncompliant code example encrypts the map into a SealedObject
, rendering the data inaccessable to prying eyes. However, since the data is not signed, it provides no proof of authentication.
Code Block | ||
---|---|---|
| ||
public static void main(String[] args)
throws IOException, GeneralSecurityException, ClassNotFoundException {
// Build map
SerializableMap< String, Integer> map = buildMap();
// Generate sealing key & seal map
KeyGenerator generator;
generator = KeyGenerator.getInstance("DES");
generator.init(new SecureRandom());
Key key = generator.generateKey();
Cipher cipher = Cipher.getInstance("DES");
cipher.init( Cipher.ENCRYPT_MODE, key);
SealedObject sealedMap = new SealedObject( map, cipher);
// Serialize map
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data"));
out.writeObject( sealedMap);
out.close();
// Deserialize map
ObjectInputStream in = new ObjectInputStream(new FileInputStream("data"));
sealedMap = (SealedObject) in.readObject();
in.close();
// Unseal map
cipher = Cipher.getInstance("DES");
cipher.init( Cipher.DECRYPT_MODE, key);
map = (SerializableMap< String, Integer>) sealedMap.getObject(cipher);
// Inspect map
InspectMap( map);
}
|
Noncompliant Code Example (Seal, Sign)
Use In addition, use the java.security.SignedObject
class to sign the an object, when the integrity of the object is to be ensured. The two new arguments passed in to the SignedObject()
method to sign the object are Signature
and a private key derived from a KeyPair
object. To verify the signature, a PublicKey
as well as a Signature
argument is passed to the SignedObject.verify()
method. This enables the code to comply with SEC17-J. Create and sign a SignedObject before creating a SealedObject
This noncompliant code example signs the object as well as sealing it. Unfortunately, the signing occurs after the sealing. As discussed above, anyone can sign a sealed object, and so it cannot be assumed that the signer is the true originator of the object.
Code Block | |||
---|---|---|---|
| |||
public static void main(String[] args) throws IOException, GeneralSecurityException, ClassNotFoundException { // Build map SerializableMap< String, Integer> map = buildMap(); // Generate sealing key & seal map KeyGenerator generator; generator = KeyGenerator.getInstance("DES"); generator.init(new SecureRandom()); Key key = generator.generateKey(); Cipher cipher = Cipher.getInstance("DES"); cipher.init( Cipher.ENCRYPT_MODE, key); SealedObject sealedMap = new SealedObject( map, cipher); // Generate signing public/private key pair & sign map KeyPairGenerator kpg = KeyPairGenerator.getInstance("DSA"); KeyPair kp = kpg.generateKeyPair(); Signature sig = Signature.getInstance("SHA1withDSA"); SignedObject class SerializableMap<K,V> implements Serializable { // other fields and methods... private SignedObject signedMap; public void sign(Signature sig, PrivateKey key) throws IOException, GeneralSecurityException { signedMap = new SignedObject( mapsealedMap, keykp.getPrivate(), sig); // Serialize map ObjectOutputStream out = null new ObjectOutputStream(new FileOutputStream("data")); out.writeObject( signedMap); } out.close(); // Deserialize map publicObjectInputStream voidin unsign(Signature sig, PublicKey key)= new ObjectInputStream(new FileInputStream("data")); signedMap throws IOException, GeneralSecurityException, ClassNotFoundException { = (SignedObject) in.readObject(); in.close(); // Unsign map if (!signedMap.verify(kp.getPublic(key), sig)) { throw new GeneralSecurityException("Map failed verification"); } mapsealedMap = (HashMap<K,V>SealedObject) signedMap.getObject(); // Unseal map signedMapcipher = nullCipher.getInstance("DES"); cipher.init( } } private SealedObject sealedMap; public void seal(Cipher cipher) Cipher.DECRYPT_MODE, key); map = (SerializableMap< String, Integer>) sealedMap.getObject(cipher); // Inspect map InspectMap( map); } |
Compliant Solution (Sign, Seal)
This compliant solution correctly signs the object before sealing it. This provides a guarantee of authenticity to the object, in addition to protection from man-in-the-middle attacks.
Code Block | ||
---|---|---|
| ||
public static void main(String[] args) throws IOException, IllegalBlockSizeExceptionGeneralSecurityException, ClassNotFoundException { // Build sealedMapmap = newSerializableMap< SealedObject(signedMap, cipherString, Integer> map = buildMap(); // Generate signing public//private Nowkey setpair the& Mapsign tomap null soKeyPairGenerator thatkpg original data does not remain in cleartext signedMap = null; } public void unseal(Cipher cipher) throws IOException, GeneralSecurityException, ClassNotFoundException { = KeyPairGenerator.getInstance("DSA"); KeyPair kp = kpg.generateKeyPair(); Signature sig = Signature.getInstance("SHA1withDSA"); SignedObject signedMap = new SignedObject( map, kp.getPrivate(), sig); // Generate sealing key & seal map KeyGenerator generator; generator = KeyGenerator.getInstance("DES"); generator.init(new SecureRandom()); Key key = generator.generateKey(); Cipher cipher = Cipher.getInstance("DES"); cipher.init( Cipher.ENCRYPT_MODE, key); SealedObject sealedMap = new SealedObject( signedMap, cipher); // Serialize map ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data")); out.writeObject( sealedMap); out.close(); // Deserialize map ObjectInputStream in = new ObjectInputStream(new FileInputStream("data")); sealedMap = (SealedObject) in.readObject(); in.close(); // Unseal map cipher = Cipher.getInstance("DES"); cipher.init( Cipher.DECRYPT_MODE, key); signedMap = (SignedObject) sealedMap.getObject(cipher); // Unsign map if (!signedMap.verify(kp.getPublic(), sig)) { throw new GeneralSecurityException("Map sealedMap = null; } } failed verification"); } map = (SerializableMap<String, Integer>) signedMap.getObject(); // Inspect map InspectMap( map); } |
Exceptions
Wiki Markup |
---|
*SEC16-EX0:* A reasonable use for signing a sealed object is to certify the authenticity of a sealed object passed from elsewhere. In the spirit of the \[[Abadi 1996|AA. Bibliography#Abadi 96]\] quotation above, this represents a commitment _about the sealed object itself_ rather than about its content. |
Risk Assessment
Failure to sign and/or seal objects during transit can lead to loss of object integrity or confidentiality.
...