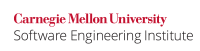
Sometimes, when Programmers sometimes misconstrue that declaring a variable is declared final
, it is believed to be final
makes the referenced object immutable. If the variable is a primitive type, declaring it final
means that its value cannot be changed after initialization (other than through the use of the unsupported sun.misc.Unsafe
class).
However, if the variable is a reference refers to a mutable object, the object's members that appear to be immutable, may in fact be mutable. For example, a final
field that stores a reference to an object does not imply immutability of the object itself. Similarly, a final
method parameter obtains a copy of the object reference through pass-by-value , but the referenced data remains mutable.
...
In this noncompliant code example, the values of instance fields a
x
and b
y
can be changed even after their initialization . When an object reference is declared final
, it only signifies that the reference cannot be changed, whereas the referenced contents can still be altereddespite the point
instance being declared as final
.
Code Block | ||
---|---|---|
| ||
class FinalClassPoint { private int ax; private int by; FinalClassPoint(int ax, int by){ this.ax = ax; this.by = by; } void set_abxy(int ax, int by){ this.ax = ax; this.by = by; } void print_abxy(){ System.out.println("the value ax is: " + this.ax); System.out.println("the value by is: " + this.by); } } public class FinalCallerPointCaller { public static void main(String[] args) { final FinalClassPoint fcpoint = new FinalClassPoint(1, 2); fcpoint.print_abxy(); // change the value of ax,b. y fcpoint.set_abxy(5, 6); fcpoint.print_abxy(); } } |
When an object reference is declared final
, it only signifies that the reference cannot be changed, whereas the referenced contents can still be altered.
Compliant Solution (final
fields)
If a
x
and b
y
must remain immutable after their initialization, they should be declared as final
. However, this requires the elimination of the setter method set_abxy()
.
Code Block | ||
---|---|---|
| ||
class FinalClassPoint { private final int ax; private final int y; Point(int b x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); // System.out.println("the value y is: " + this.y); } } |
Compliant Solution (provide copy functionality)
This compliant solution provides a clone()
method in the final
class Point
and does not require the elimination of the setter method.
Code Block | ||
---|---|---|
| ||
final public class FinalClassPoint implements Cloneable { private int ax; private int y; Point(int b; FinalClass(int a x, int y){ this.x = x; this.y = y; } void set_xy(int x, int by){ this.ax = ax; this.by = by; } void print_abxy(){ System.out.println("the value ax is: "+ this.ax); System.out.println("the value by is: "+ this.by); } void set_ab(int a, int b)public Point clone() throws CloneNotSupportedException{ this.aPoint cloned = a(Point) super.clone(); thiscloned.bx = b; } public FinalClass clone() throws CloneNotSupportedException{this.x; FinalClass cloned.y = (FinalClass) super.clone()this.y; return cloned; } } public class FinalCallerPointCaller { public static void main(String[] args) throws CloneNotSupportedException { final FinalClassPoint nfpoint = new FinalClassPoint(1, 2); nfpoint.print_abxy(); // Get the copy of original object Point pointCopy FinalClass nf2 = nfpoint.clone(); // Change the value of ax,by of the copy. nf2pointCopy.set_abxy(5, 6); // Original value will not be changedremains unchanged nfpoint.print_abxy(); } } |
The clone()
method returns a copy of the original object and reflects its latest state. This new object can be freely used without exposing the original object. Using the clone()
method allows the class to remain mutable. (OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely)
The FinalClass
Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object. This compliant solution complies with OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.
Noncompliant Code Example (arrays)
...
This noncompliant code example uses a {{public
static
final
}} array. Clients can trivially modify the contents of the array (although they are unable to change the array reference, as it is {{final}}). In this noncompliant code example, the elements of the {{items
\[\]}} array, are modifiable.
Code Block | ||
---|---|---|
| ||
public static final String[] items = { ... }; |
Clients can trivially modify the contents of the array, though they are unable to change the array reference because it is final
.
Compliant Solution (clone the array)
...
As a result, the original array values cannot be modified by a client. Note that sometimes, a manual deep copy may be required when dealing with arrays of objects. This generally happens when the objects do not export a clone()
method. Refer to FIO00-J. Defensively copy mutable inputs and mutable internal components for more information.
Compliant Solution (unmodifiable wrappers)
...