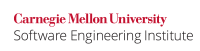
...
In this compliant solution, each BankAccount
has a java.util.concurrent.locks.ReentrantLock
associated with it. This permits the depositAllAmount()
method to try acquiring both accounts' locks, but releasing its locks if it fails, and trying again later.
Code Block | ||
---|---|---|
| ||
class BankAccount { private int balanceAmount; // Total amount in bank account private final Lock lock = new ReentrantLock(); Lock getLock() { return lock; } private static final int TIME = 1000; // 1 mssecond private BankAccount(int balance) { this.balanceAmount = balance; } // Deposits the amount from this object instance to BankAccount instance argument ba private void depositAllAmount(BankAccount ba) throws InterruptedException { while (true) { if (this.lock.tryLock()) { try { if (ba.lock.tryLock()) { try { ba.balanceAmount += this.balanceAmount; this.balanceAmount = 0; // withdraw all amount from this instance ba.displayAllAmount(); // Display the new balanceAmount in ba (may cause deadlock) break; } finally { ba.getLock().unlock(); } } } finally { this.getLock().unlock(); } } Thread.sleep( TIME); } } private void displayAllAmount() throws InterruptedException { while (true) { if (lock.tryLock()) { try { System.out.println(balanceAmount); break; } finally { lock.unlock(); } } Thread.sleep( TIME); } } public static void initiateTransfer(final BankAccount first, final BankAccount second) { Thread t = new Thread(new Runnable() { public void run() { first.depositAllAmount(second); } }); t.start(); } } |
...