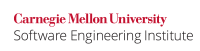
...
Code Block | ||
---|---|---|
| ||
class DBConnector implements Runnable {
final String query;
DBConnector(String query) {
this.query = query;
}
public void run() {
Connection con;
try {
// Username and password are hard coded for brevity
con = DriverManager.getConnection("jdbc:myDriver:name", "username","password");
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery(query);
} catch (SQLException e) {
// Forward to handler
}
// ...
}
}
|
...
Code Block | ||
---|---|---|
| ||
class DBConnector implements Runnable {
final String query;
DBConnector(String query) {
this.query = query;
}
private static ThreadLocal<Connection> connectionHolder = new ThreadLocal<Connection>() {
Connection connection = null;
@Override public Connection initialValue() {
try {
// Username and password are hard coded for brevity
connection = DriverManager.getConnection
("jdbc:driver:name", "username","password");
} catch (SQLException e) {
// Forward to handler
}
return connection;
}
@Override public void set(Connection con) {
if(connection == null) { // Shuts down socket when null value is passed
try {
connection.close();
} catch (SQLException e) {
// Forward to handler
}
} else {
connection = con;
}
}
};
public static Connection getConnection() {
return connectionHolder.get();
}
public static void shutdownConnection() { // Allows client to close socket anytime
connectionHolder.set(null);
}
public void run() {
Connection dbConnection = getConnection();
Statement stmt;
try {
stmt = dbConnection.createStatement();
ResultSet rs = stmt.executeQuery(query);
} catch (SQLException e) {
// Forward to handler
}
// ...
}
}
|
...