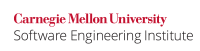
...
Code Block | ||
---|---|---|
| ||
public int compare(Integer i, Integer j) { return i < j ? -1 : (i > j ? 1 : 0) ; } |
Noncompliant Code Example
Sometimes a list of integers is desired. Recall that the type parameter inside the angle brackets of a list cannot be of a primitive type. It is not possible to form an ArrayList<int>
. With the help of the wrapper classs and autoboxing, storing primitive integer values in ArrayList<Integer>
becomes possible.
In this noncompliant code example, it is desired to count the integers of arrays list1
and list2
. As class Integer
can only cache integers from -127 to 128, when an int
number is beyond this range, it is autoboxed into the corresponding wrapper type. The ==
operator returns false
when these distinct wrapper objects are compared. As a result, the output of this example is 0.
Code Block | ||
---|---|---|
| ||
import java.util.ArrayList;
public class Wrapper {
public static void main(String[] args) {
// Create an array list of integers, where each element
// is greater than 127
ArrayList<Integer> list1 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list1.add(i+1000);
// Create another array list of integers, where each element
// is the same as the first list
ArrayList<Integer> list2 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list2.add(i+1000);
int counter = 0;
for(int i=0;i<10;i++)
if(list1.get(i) == list2.get(i))
counter++;
// print the counter
System.out.println(counter);
}
}
|
If it were possible to expand the cache inside Integer
(cache all the integer values -32K-32K, which means that all the int
values may be autoboxed to the same Integer
object), then the results may have differed.
Compliant Solution
This compliant solution uses the equals()
method for performing comparisons of wrapped objects. It produces the correct output 10.
Code Block | ||
---|---|---|
| ||
public class TestWrapper1 {
public static void main(String[] args) {
// Create an array list of integers, where each element
// is greater than 127
ArrayList<Integer> list1 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list1.add(i+1000);
// Create another array list of integers, where each element
// is the same as the first one
ArrayList<Integer> list2 = new ArrayList<Integer>();
for(int i=0;i<10;i++)
list2.add(i+1000);
int counter = 0;
for(int i=0;i<10;i++)
if(list1.get(i).equals(list2.get(i))) counter++;
System.out.println(counter);
}
}
|
Risk Assessment
Using the equal and not equal operators to compare boxed primitives can lead to erroneous comparisons.
...