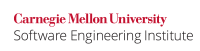
Every declaration should be for a single variable, on its own line, with an explanatory comment about the role of the variable. Declaring multiple variables in a single declaration can cause confusion regarding the types of the variables and their initial values. If more than one variable is declared in a declaration, care must be taken to ensure that the type and initialized value of the variable is knownself evident.
Noncompliant Code Example
Wiki Markup |
---|
In this noncompliant code example, a programmer or code reviewer might mistakenly believeconceive that the two variables {{src}} and {{c}} are declared as {{int}}. In fact, {{src}} hasis aof type of {{int \[\]}}, while {{c}} has a type of {{int}}. |
Code Block | ||
---|---|---|
| ||
int src[], c;
|
Another fallout of this example is that it declares the array in a largely antiquated and unpopular style, with the brackets appearing after the variable name as in type name[]
. In practice, arrays are typically declared as type[] name
.
Compliant Solution
In this compliant solution, each variable is declared on a separate line. It also uses the preferable style for declaring arrays.
Code Block | ||
---|---|---|
| ||
int[] src[]; /* source array */ int c; /* max value */ |
...
In this noncompliant example, a programmer or code reviewer might mistakenly believe that both i
and j
have been initialized to 1. In fact, only j
has been initialized, while i
remains uninitialized.
Code Block | ||
---|---|---|
| ||
int i, j = 1;
|
Compliant Solution
In this compliant solution, it is readily apparent that both i
and j
have been initialized to 1.
Code Block | ||
---|---|---|
| ||
int i = 1;
int j = 1;
|
Nomcompliant Example
In this noncompliant example, the original programmer declared multiple variables, including an array, on the same line. Since even arrays All instances of the type T
have access to all Object
methods, mistakes of this form may not be immediately detected by the compiler or an IDEmethods of the class Object
. However, it is easy to miss that arrays need special treatment when some of these methods are overridden. Oversights of this genre typically go undetected by compilers and IDEs, alike.
Code Block | ||
---|---|---|
| ||
public class Example{ private T a,b,c[],d; public Example(T in){ a = in; b = in; c = (T[]) new Object[10]; d = in; } |
Thus, when it comes time to write something like the toString
methoda method of Object
like toString()
is overridden, a programmer might accidentally write it provide a general implementation for type T
without realizing that c
is an array. Since the mistake compiles cleanly, it may go undetected.
Code Block |
---|
// The oversight error leads to an incorrect implementation
|
No Format |
public String toString(){
return a.toString() + b.toString() + c.toString() + d.toString();
}
|
However, the intended toString
real intent might have been to invoke toString
for each T
in ()
on each individual member of the type T
, in array c
.
Code Block |
---|
// Correct functional implementation
|
No Format |
public String toString(){
String s = a.toString() + b.toString();
for(int i = 0; i < c.length; i++){
s += c[i].toString();
}
s += d.toString();
return s;
}
|
Compliant Solution
Move To be compliant, move each declaration to a different line, so programmer error of thinking c
is a T
object, isn't as likely. Furthermore, declare arrays by putting placing the brackets adjacent to the type, as opposed to postfixed to the variable nameusing the postfix notation.
Code Block | ||
---|---|---|
| ||
public class Example { private T a; private T b; private T[] c; private T d; public Example(T in){ a = in; b = in; c = (T[]) new Object[10]; d = in; } } |
Exceptions
DCL04-01: Trivial declarations for loop counters, for example, can reasonably be included within a for
statement:
Code Block | ||
---|---|---|
| ||
for (int i = 0; i < mx; ++i ) {
/* ... */
}
|
Risk Assessment
Declaring Failing to declare no more than one variable per declaration can make affect code easier to read and eliminate confusionreadability and cause misinterpretations.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL04-J | low | unlikely | low | P3 | L3 |
...