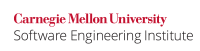
...
The erroneous behavior is caused due to the server returning null
while the client forgets to add in a check for such a value. This noncompliant example shows how the check item != null
condition is missing from the if
condition in class Client
.
Code Block | ||
---|---|---|
| ||
import java.util.Arrays;
class Inventory {
private static int[] item;
public Inventory() {
item = new int[20];
}
public static int[] getStock() {
if(item.length == 0)
return null;
else
return item;
}
}
public class Client {
public static void main(String[] args) {
Inventory iv = new Inventory();
int[] item = Inventory.getStock();
if (Arrays.asList(item[1]).contains(1)) {
System.out.println("Almost out of stock!" + item);
}
}
}
|
...
Wiki Markup |
---|
This compliant solution eliminates the {{null}} return and simply returns the {{item}} array as is even if it is zero-length. The client can effectively handle this situation without exhibiting erroneous behavior. Be careful that the client does not try to access individual elements of a zero-length array such as {{item\[1\]}} while following this recommendation. |
Code Block | ||
---|---|---|
| ||
import java.util.Arrays; class Inventory { private static int[] item; public Inventory() { item = new int[20]; item[2] = 1; //quantity of item 2 remaining is 1, almost out! } public static int[] getStock() { return item; } } public class Client { public static void main(String[] args) { Inventory iv = new Inventory(); int[] item = Inventory.getStock(); if (Arrays.asList(item[1]).contains(1)) { System.out.println("Almost out of stock!" + item); } } } |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET03-J | low | unlikely | high | P1 | L3 |
Automated Detection
TODO
Other Languages
This guideline appears in the C Secure Coding Standard as MSC19-C. For functions that return an array, prefer returning an empty array over a null value.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...