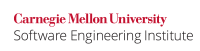
...
The final
keyword in Java is used to declare constants. Its effect is to render the affected variable immutable. Attempting to change the value of a final
-qualified variable results in a compile-time error. Since constants cannot be changed, it is desirable to define only one instance of them for the class; hence constants should be declared with the static
modifier as well.
The following code fragment demonstrates its usethe use of static
and final
to create a constant:
Code Block |
---|
static final int SIZE=25;
|
This code declares the value SIZE to be of type int and to have the immutable value 25. This constant can subsequently be used whenever the value 25 would be needed.
...
In this compliant solution, a constant PI is first declared and set equal to 3.14, and is thereafter referenced in the code whenever the value pi is needed.
Code Block | ||
---|---|---|
| ||
static final int PI = 3.14;
double area(double radius){
return 4.0*PI*radius*radius;}
double volume(double radius){
return 4.0/3.0*PI*radius*radius*radius;}
double greatCircleCircumference(double radius){
return 2*PI*radius;}
|
This both clarifies the code and allows easy editing, for if a different value for pi is required, the programmer can simply redefine the constant.
Compliant Solution: Predefined Constants
Java.lang.Math defines a large set of numeric constants, such as java.lang.Math.PI and java.lang.Math.E. If such constants exists, it is preferable to use them, rather than redefining the constant yourself.
Code Block | ||
---|---|---|
| ||
double area(double radius){
return 4.0*Math.PI*radius*radius;}
double volume(double radius){
return 4.0/3.0*Math.PI*radius*radius*radius;}
double greatCircleCircumference(double radius){
return 2*Math.PI*radius;}
|
Exceptions
The use of symbolic constants should be restricted to cases where they improve the readability and maintainability of the code. Using them when the intent of the literal is obvious, or where the literal is not likely to change, can impair code readability. In the Compliant Solution above, the values 4.0 and 3.0 in the volume calculation are clearly scaling factors used to calculate the circle volume, and as such are not subject to change (unlike pi, they can be represented exactly, so there is no reason to change them to increase precision). Hence, replacing them with symbolic constants would be inappropriate.
...