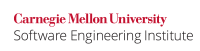
The conditional operator ?:
uses the boolean
value of one expression to decide which of the other two expressions should be evaluated. (see See JLS, Section 15.25, "Conditional Operator ? :
".) .
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
- If the value of the first operand (
operand1
) istrue
, then the second operand expression (operand2
) is chosen. - If the value of the first operand is
false
, then the third operand expression (operand3
) is chosen.
The conditional operator is syntactically right-associative; for example, a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The JLS-defined rules for determining the type of the result of a conditional expression (tabulated below) are quite complicated; programmers may could be surprised by the type conversions required for expressions they have written.
...
Operand 2 | Operand 3 | Resultant type |
---|---|---|
type T | type T | type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric | other numeric | promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) | T2 = boxing conversion(S2) | apply capture conversion to lub(T1,T2) |
See JLS, Section 5.1.7, "Boxing Conversion,"; JLS, Section 5.1.10, "Capture Conversion"; and JLS, Section 15.12.2.7, "Inferring Type Arguments Based on Actual Arguments" for additional information on the final table entry.
...
This noncompliant code example prints the value of alpha
as A
, which is of the char
type. The third operand is a constant expression of type int
, whose value 0
can be represented as a char
; numeric promotion is unnecessary. However, this behavior depends on the particular value of the constant integer expression; changing that value may can lead to different behavior.
...
This noncompliant example prints 65
— the {{65}}â”the ASCII equivalent of A
— , instead of the expected A
, because the second operand (alpha
) must be promoted to type int
. The numeric promotion occurs because the value of the third operand (the constant expression '12345') is too large to be represented as a char
.
...
The compliant solution explicitly states the intended result type by casting alpha
to type int
. Casting 12345
to type char
would ensure that both operands of the conditional expression have the same type, and would result resulting in A
being printed. However, it would result in data loss when an integer larger than Character.MAX_VALUE
is downcast to type char
. This compliant solution avoids potential truncation by casting alpha
to type int
, the wider of the operand types.
...
When the second and third operands of a conditional expression have different types, they may can be subject to type conversions that were not anticipated by the programmer.
...