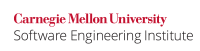
...
Result type determination begins from the top of the table; the compiler applies the first matching rule. The table refers to constant expressions of type int
(such as '0' or variables declared final
) as constant int
; the "Operand 2" and "Operand 3" columns refer to operand2
and operand3
(from the above definition), respectively. In the table, constant int
refers to constant expressions of type int
(such as '0' or variables declared final
).
Operand 2 | Operand 3 | Resultant type | ||
---|---|---|---|---|
type T | type T | type T | ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
|
|
|
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
|
|
| ||
other numeric | other numeric | promoted type of the 2nd and 3rd operands | ||
T1 = boxing conversion (S1) | T2 = boxing conversion(S2) | apply capture conversion to lub(T1,T2) |
...
This noncompliant example prints {{65}}â”the ASCII equivalent of A
, instead of the expected A
, because the second operand (alpha
) must be promoted to type int
. The numeric promotion occurs because the value of the third operand (the constant expression '12345123456') is too large to be represented as a char
.
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 12345123456); } } |
Compliant Solution
The compliant solution explicitly states the intended result type by casting alpha
to type int
. Casting 12345
123456
to type char
would ensure that both operands of the conditional expression have the same type, resulting in A
being printed. However, it would result in data loss when an integer larger than Character.MAX_VALUE
is downcast to type char
. This compliant solution avoids potential truncation by casting alpha
to type int
, the wider of the operand types.
Code Block | ||
---|---|---|
| ||
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast alpha as an int to explicitly state that the type of the // conditional expression should be int. System.out.print(true ? ((int) alpha) : 12345123456); } } |
Noncompliant Code Example
...