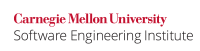
...
Code Block | ||
---|---|---|
| ||
public class Overloader {
private static String display(ArrayList<Integer> a) {
return "ArrayList";
}
private static String display(LinkedList<String> l) {
return "LinkedList";
}
private static String display(List<?> l) {
return "List is not recognized";
}
public static void main(String[] args) {
// Single ArrayList
System.out.println(display(new ArrayList<Integer>()));
// Array of lists
List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(),
new LinkedList<String>(), new Vector<Integer>()};
for (List<?> i : invokeAll) {
System.out.println(display(i));
}
}
}
|
Wiki Markup |
---|
At compile time, the type of the object array is {{List}}. The expected output is {{ArrayList}}, {{ArrayList}}, {{LinkedList}} and {{List is not recognized}} ( because {{java.util.Vector}} does not inherit from {{java.util.List}}). However, in all The actual output is {{ArrayList}} followed by three instances of {{List is not recognized}}. The iscause displayed.of Thisthis happensunexpected becausebehavior inis overloading,that theoverloaded method invocations are notaffected affected_only_ by the runtimecompile typestime buttype onlyof thetheir compile time type (arguments: {{ListArrayList}}). Itfor isthe dangerousfirst toinvocation implement overloading to tally with overriding, more so, because the latter is characterized by inheritance unlike the formerand {{List}} for the others. Do not use overloading where overriding would be natural \[[Bloch 2008|AA. Bibliography#Bloch 08]\]. |
...
This compliant solution uses a single display
method and instanceof
to distinguish between different types. As expected, the output is ArrayList
, ArrayList
, LinkedList
, List is not recognized
.
Code Block | ||
---|---|---|
| ||
class Overloader {
public class Overloader {
private static String display(List<?> l) {
return (
l instanceof ArrayList ? "Arraylist" :
(l instanceof LinkedList ? "LinkedList" :
"List is not recognized")
);
}
public static void main(String[] args) {
// Single ArrayList
System.out.println(display(new ArrayList<Integer>()));
List<?>[] invokeAll = new List<?>[] {new ArrayList<Integer>(),
new LinkedList<String>(), new Vector<Integer>()};
for (List<?> i : invokeAll) {
System.out.println(display(i));
}
}
}
|
...
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET05-J | low | unlikely | high | P1 | L3 |
Automated Detection
TODOSound automated detection of violations is infeasible, because it would require determination of programmer intent. Heuristic techniques may be useful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
...