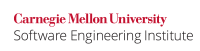
...
This noncompliant code example contains a doSomething()
method that starts a thread. The thread supports interruption by checking a volatile flag and blocks waiting until notified. The stop()
method checks to see whether the thread is blocked on the wait; if so, it sets the flag to true and notifies the thread so that the thread can terminate.
Code Block | ||
---|---|---|
| ||
public class Waiter {
private Thread thread;
private volatile boolean flag;
private final Object lock = new Object();
public void doSomething() {
thread = new Thread(new Runnable() {
@Override public void run() {
synchronized(lock) {
while (!flag) {
try {
lock.wait();
// ...
} catch (InterruptedException e) {
// Forward to handler
}
}
}
}
});
thread.start();
}
public boolean stop() {
if (thread != null) {
if (thread.getState() == Thread.State.WAITING) {
flag = true;
synchronized (lock) {
lock.notifyAll();
}
return true;
}
}
return false;
}
}
|
...