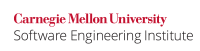
...
This noncompliant code example declares the Properties
instance Map instance field volatile. The instance of the Properties
object Map
object can be mutated using the put()
method; consequently, it is a mutable object.
Code Block | ||
---|---|---|
| ||
final class Foo { private volatile Map<String, PropertiesString> propertiesmap; public Foo() { propertiesmap = new PropertiesHashMap<String, String>(); // Load some useful values into propertiesmap } public String get(String s) { return propertiesmap.getPropertyget(s); } public void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } propertiesmap.setPropertyput(key, value); } } |
Interleaved calls to get()
and put()
may result in internally inconsistent values being retrieved from the Properties
object Map object because the operations within put()
modify its state. Declaring the object reference volatile is insufficient to eliminate this data race.
The put()
method lacks a time-of-check, time-of-use (TOCTOU) vulnerability, despite the presence of the validation logic, because the validation is performed on the immutable value
argument rather than on the shared Properties
instance Map
instance.
Noncompliant Code Example (Volatile-Read, Synchronized-Write)
This noncompliant code example attempts to use the volatile-read, synchronized-write technique described by Goetz [Goetz 2007]. The properties
field map
field is declared volatile to synchronize its reads and writes. The put()
method is also synchronized to ensure that its statements are executed atomically.
Code Block | ||
---|---|---|
| ||
final class Foo { private volatile Properties propertiesMap<String, String> map; public Foo() { propertiesmap = new PropertiesHashMap<String, String>(); // Load some useful values into propertiesmap } public String get(String s) { return propertiesmap.getPropertyget(s); } public synchronized void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } propertiesmap.setPropertyput(key, value); } } |
The volatile-read, synchronized-write technique uses synchronization to preserve atomicity of compound operations, such as increment, and provides faster access times for atomic reads. However, it does not work with mutable objects because the visibility guarantee provided by volatile
extends only to the field itself (the primitive value or object reference); the referent (and hence the referent's members) is excluded from the guarantee. Consequently, the write and a subsequent read of the property map lack a happens-before relationship.
...
Code Block | ||
---|---|---|
| ||
final class Foo { private final Map<String, PropertiesString> propertiesmap; public Foo() { propertiesmap = new PropertiesHashMap<String, String>(); // Load some useful values into propertiesmap } public synchronized String get(String s) { return propertiesmap.getPropertyget(s); } public synchronized void put(String key, String value) { // Validate the values before inserting if (!value.matches("[\\w]*")) { throw new IllegalArgumentException(); } propertiesmap.setPropertyput(key, value); } } |
It is unnecessary to declare the properties
field map field volatile because the accessor methods are synchronized. The field is declared final to prevent publication of its reference when the referent is in a partially initialized state (see rule TSM03-J. Do not publish partially initialized objects for more information).
...