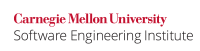
...
This noncompliant code example contains a finally
block that closes the reader
object. The programmer incorrectly assumes that the statements in the finally
block cannot throw exceptions and consequently fails to appropriately handle any exception that may arise.
Code Block | ||
---|---|---|
| ||
public class Operation {
public static void doOperation(String some_file) {
// ... code to check or set character encoding ...
try {
BufferedReader reader =
new BufferedReader(new FileReader(some_file));
try {
// Do operations
} finally {
reader.close();
// ... Other cleanup code ...
}
} catch (IOException x) {
// Forward to handler
}
}
}
|
...
This compliant solution encloses the close()
method invocation in a try-catch
block of its own within the finally
block. Consequently, the potential IOException
can be handled without allowing it to propagate further.
Code Block | ||
---|---|---|
| ||
public class Operation {
public static void doOperation(String some_file) {
// ... code to check or set character encoding ...
try {
BufferedReader reader =
new BufferedReader(new FileReader(some_file));
try {
// Do operations
} finally {
try {
reader.close();
} catch (IOException ie) {
// Forward to handler
}
// ... Other clean-up code ...
}
} catch (IOException x) {
// Forward to handler
}
}
}
|
...
Java SE 7 introduced a new feature, called try-with-resources, that can close certain resources automatically in the event of an error. This compliant solution uses try-with-resources to properly close the file.
Code Block | ||
---|---|---|
| ||
public class Operation {
public static void doOperation(String some_file) {
// ... code to check or set character encoding ...
try ( // try-with-resources
BufferedReader reader =
new BufferedReader(new FileReader(some_file))) {
// Do operations
} catch (IOException ex) {
System.err.println("thrown exception: " + ex.toString());
Throwable[] suppressed = ex.getSuppressed();
for (int i = 0; i < suppressed.length; i++) {
System.err.println("suppressed exception: "
+ suppressed[i].toString());
}
// Forward to handler
}
}
public static void main(String[] args) {
if (args.length < 1) {
System.out.println("Please supply a path as an argument");
return;
}
doOperation(args[0]);
}
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR05-J | low | unlikely | medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | PW.ABNORMAL_TERMINATION_ OF_FINALLY_BLOCK | Implemented |
Related Guidelines
CWE-460. Improper cleanup on thrown exception | |
| CWE-584. Return inside |
| CWE-248. Uncaught exception |
| CWE-705. Incorrect control flow scoping |
...
Puzzle 41. Field and Stream | |
8.3, Preventing Resource Leaks (Java) | |
| |
The try-with-resources Statement |