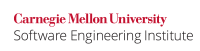
...
In this noncompliant code example, the finally
block completes abruptly because of a return
statement in the block.
Code Block | ||
---|---|---|
| ||
class TryFinally {
private static boolean doLogic() {
try {
throw new IllegalStateException();
} finally {
System.out.println("logic done");
return true;
}
}
}
|
...
This compliant solution removes the return
statement from the finally
block.
Code Block | ||
---|---|---|
| ||
class TryFinally {
private static boolean doLogic() {
try {
throw new IllegalStateException();
} finally {
System.out.println("logic done");
}
// Any return statements must go here;
// applicable only when exception is thrown conditionally
}
}
|
...
For example, the following code does not violate this rule, because the break
statement exits the while
loop, but not the finally
block.
Code Block | ||
---|---|---|
| ||
class TryFinally {
private static boolean doLogic() {
try {
throw new IllegalStateException();
} finally {
int c;
try {
while ((c = input.read()) != -1) {
if (c > 128) {
break;
}
}
} catch (IOException x) {
// forward to handler
}
System.out.println("logic done");
}
// Any return statements must go here; applicable only when exception is thrown conditionally
}
}
|
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR04-J | low | probable | medium | P4 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Coverity | 7.5 | PW.ABNORMAL_TERMINATION_ OF_FINALLY_BLOCK | Implemented |
Related Guidelines
...
Puzzle 36. Indecision | |
8.2, Managing Exceptions, The Vanishing Exception | |
[JLS 2005] |