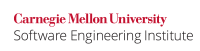
...
This noncompliant code example casts the value returned by the read()
method directly to a value of type byte
and then compares this value with -1 in an attempt to detect the end of the stream.
Code Block | ||
---|---|---|
| ||
FileInputStream in;
// initialize stream
byte data;
while ((data = (byte) in.read()) != -1) {
// ...
}
|
...
Use a variable of type int
to capture the return value of the byte
input method. When the value returned by read()
is not -1, it can be safely cast to type byte
. When read()
returns 0x000000FF
, the comparison will test against 0xFFFFFFFF
, which evaluates to false
.
Code Block | ||
---|---|---|
| ||
FileInputStream in;
// initialize stream
int inbuff;
byte data;
while ((inbuff = in.read()) != -1) {
data = (byte) inbuff;
// ...
}
|
...
This noncompliant code example casts the value of type int
returned by the read()
method directly to a value of type char
, which is then compared with -1 in an attempt to detect the end of stream. This conversion leaves the value of data
as 0xFFFF
(e.g., Character.MAX_VALUE
) instead of -1. Consequently, the test for the end of file never evaluates to true.
Code Block | ||
---|---|---|
| ||
FileReader in;
// initialize stream
char data;
while ((data = (char) in.read()) != -1) {
// ...
}
|
...
Use a variable of type int
to capture the return value of the character input method. When the value returned by read()
is not -1, it can be safely cast to type char
.
Code Block | ||
---|---|---|
| ||
FileReader in;
// initialize stream
int inbuff;
char data;
while ((inbuff = in.read()) != -1) {
data = (char) inbuff;
// ...
}
|
...
Related Guidelines
VOID FIO34-CPP. Use int to capture the return value of character IO functions |
Bibliography