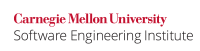
...
This technique is also called client-side locking [Goetz 2006] because the class holds a lock on an object that might be accessible to other classes. Client-side locking is not always an appropriate strategy; see rule LCK11-J. Avoid client-side locking when using classes that do not commit to their locking strategy for more information.
...
This noncompliant code example defines the KeyedCounter
class that is not thread-safe. Although the HashMap
is wrapped in a synchronizedMap()
, the overall increment operation is not atomic [Lee 2009].
Code Block | ||
---|---|---|
| ||
final class KeyedCounter { private final Map<String, Integer> map = Collections.synchronizedMap(new HashMap<String, Integer>()); public void increment(String key) { Integer old = map.get(key); int oldValue = (old == null) ? 0 : old.intValue(); if (oldValue == Integer.MAX_VALUE) { throw new ArithmeticException("Out of range"); } map.put( key, oldValue + 1); } public Integer getCount(String key) { return map.get(key); } } |
...
The ConcurrentHashMap
class used in this compliant solution provides several utility methods for performing atomic operations and is often a good choice for algorithms that must scale [Lee 2009].
Note that this compliant solution still requires synchronization, because without it, the test to prevent overflow and the increment will not happen atomically, so two threads calling increment()
can still cause overflow. The synchronization block is smaller, and does not include the lookup or addition of new values, so it has less of an impact on performance as the previous compliant solution.
...
According to Section 5.2.1., "ConcurrentHashMap" of the work of Goetz and colleagues [Goetz 2006]:
ConcurrentHashMap
, along with the other concurrent collections, further improve on the synchronized collection classes by providing iterators that do not throwConcurrentModificationException
, as a result eliminating the need to lock the collection during iteration. The iterators returned byConcurrentHashMap
are weakly consistent instead of fail-fast. A weakly consistent iterator can tolerate concurrent modification, traverses elements as they existed when the iterator was constructed, and may (but is not guaranteed to) reflect modifications to the collection after the construction of the iterator.
...
CWE-362. Concurrent execution using shared resource with improper synchronization ("race condition") | |
| CWE-366. Race condition within a thread |
| CWE-662. Improper synchronization |
Bibliography
[API 2006] |
|
Section 4.4.1, Client-side Locking | |
| Section 5.2.1, ConcurrentHashMap |
Section 8.2, Synchronization and Collection Classes | |
[Lee 2009] | Map & Compound Operation |
...