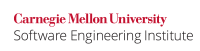
Programmers sometimes misconstrue incorrectly believe that declaring a field or variable final
makes the referenced object immutable. When the variable is Declaring variables that have a primitive type , declaring it final
means that its value cannot be changed to be final
indeed prevents changes to their values after initialization (other than through the use of the unsupported sun.misc.Unsafe
class). However, when the variable refers to a mutable object, the object's members that appear to be immutable, When the variable has a reference type, however, the presence or absence of a final
clause in the declaration makes the reference itself immutable; the final
clause lacks any effect whatsoever on the referenced object. Consequently, the fields of the referenced object could, in fact, be mutable. Similarly, a final
method parameter obtains a an immutable copy of the object reference through pass-by-value, but ; once again, this lacks any effect on the mutability of the referenced data remains mutable.
Wiki Markup |
---|
According to the Java Language Specification \[[JLS 2005|AA. Bibliography#JLS 05]\], [Section 4.12.4|http://java.sun.com/docs/books/jls/third_edition/html/typesValues.html#4.12.4], "{{final}} Variables" |
... if a
final
variable holds a reference to an array, then the components of the array may be changed by operations on the array, but the variable will always refer to the same array.
Noncompliant Code Example (Mutable Class, final
Reference)
In this noncompliant code example, the values of instance fields x
and y
can be changed even after their initialization, despite even though the reference to the point
instance being is declared to be final
.
Code Block | ||
---|---|---|
| ||
class Point { private int x; private int y; Point(int x, int y){ this.x = x; this.y = y; } void set_xy(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } public class PointCaller { public static void main(String[] args) { final Point point = new Point(1, 2); point.print_xy(); // change the value of x, y point.set_xy(5, 6); point.print_xy(); } } |
When an object reference is declared final
, it signifies only that the reference cannot be changed; the mutability of the referenced contents can still be alteredremains unaffected.
Compliant Solution (final
Fields)
When the values of the x
and y
members must remain immutable after their initialization, they should be declared final
. However, this requires the elimination of the setter method set_xy()
.
Code Block | ||
---|---|---|
| ||
class Point { private final int x; private final int y; Point(int x, int y){ this.x = x; this.y = y; } void print_xy(){ System.out.println("the value x is: " + this.x); System.out.println("the value y is: " + this.y); } } |
Compliant Solution (Provide Copy Functionality)
This compliant solution provides a clone()
method in the final
class Point
and does not require the elimination of the setter method.
...
The clone()
method returns a copy of the original object and reflects its latest state at the moment of clonging. This new object can be freely used without exposing the original object. Using the clone()
method allows the class to remain mutable. (See guideline OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.)
The Point
class is declared final
to prevent subclasses from overriding the clone()
method. This enables the class to be suitably used without any inadvertent modifications of the original object. This compliant solution complies with guideline OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely.
Noncompliant Code Example (Arrays)
This noncompliant code example uses a public static final
array, items
.
...
Clients can trivially modify the contents of the array, though, they are unable to change even though declaring the array reference because it is final
to be final
prevents modification of the reference itself.
Compliant Solution (Clone the Array)
This compliant solution defines a private
array and a public
method that returns a copy of the array.
...
Consequently, the original array values cannot be modified by a client. Note that a manual deep copy could be required when dealing with arrays of objects. This generally happens when the objects do not export a clone()
method. Refer to guideline FIO00-J. Defensively copy mutable inputs and mutable internal components for more information.
Compliant Solution (Unmodifiable Wrappers)
This compliant solution declares a private
array from which a public
immutable list is constructed.
...
Neither the original array values nor the public
list can be modified by a client. For more details about unmodifiable wrappers, refer to guideline SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers.
Risk Assessment
Using final
references to declare the reference to a mutable object is potentially misleading mutable objects can mislead unwary programmers because the contents of the object can still be changedreferenced object remain mutable.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ01-J | low | probable | medium | P4 | L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
MITRE CWE: CWE-607 "Public Static Final Field References Mutable Object"
Bibliography
Wiki Markup |
---|
\[[Bloch 2008|AA. Bibliography#Bloch 08]\] Item 13: Minimize the accessibility of classes and members \[[Core Java 2004|AA. Bibliography#Core Java 04]\] Chapter 6 \[[JLS 2005|AA. Bibliography#JLS 05]\] Sections [4.12.4 "final Variables"|http://java.sun.com/docs/books/jls/third_edition/html/typesValues.html#4.12.4] and [6.6 "Access Control"|http://java.sun.com/docs/books/jls/third_edition/html/names.html#6.6] |
...