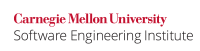
Wiki Markup |
---|
Classes that overrides the {{Object.equals()}} method must also override the {{Object.hashCode()}} method. The Java {{java.lang.Object}} class requires that any two objects that compare equal using the {{equals(Object)}} method must produce the same integer result when the {{hashCode()}} method is invoked on the objects \[[API 2006|AA. Bibliography#API 06]\]. |
...
This noncompliant code example stores a <credit card number, string> pair into associates credit card numbers with strings using a HashMap
and subsequently attempts to retrieve the string value associated with the a credit card number. The expected retrieved value is Java
; the actual retrieved value is null
. The cause of this erroneous behavior is that the CreditCard
class overrides the equals()
method but fails to override the hashCode()
method. Consequently, the default hashCode()
method returns a different value for each object, even though the objects are logically equivalent; these differing values lead to examination of different hash buckets, which prevents the get()
method from finding the intended value.
Code Block | ||
---|---|---|
| ||
public final class CreditCard {
private final int number;
public CreditCard(int number) {
this.number = (short) number;
}
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof CreditCard)) {
return false;
}
CreditCard cc = (CreditCard)o;
return cc.number == number;
}
public static void main(String[] args) {
Map<CreditCard, String> m = new HashMap<CreditCard, String>();
m.put(new CreditCard(100), "Java");
// Assuming Integer.MAX_VALUE is the largest number for card
System.out.println(m.get(new CreditCard(100)));
}
}
|
...