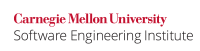
...
Programmers may confuse hiding with overriding resulting in unexpected behavior. An instance method defined in a subclass overrides another instance method in the superclass when both have the same name, number and type of parameters, and the return type.
...
In this noncompliant example, the programmer attempts to override a static method but fails to understand that the the static method has been hiddenhides the static method instead of overriding it. Consequently, his the code unexpectedly invokes the displayAccountStatus()
method of the superclass at two different call sites, rather than invoking the superclass method at one call site and the subclass method at the other . Additionally, his code uses expressions that are typical of dynamic dispatch, further indicating his confusionas the programmer likely intended.
Code Block | ||
---|---|---|
| ||
class GrantAccess { public static void displayAccountStatus() { System.out.print("Account details for admin: XX"); } } class GrantUserAccess extends GrantAccess { public static void displayAccountStatus() { System.out.print("Account details for user: XX"); } } public class StatMethod { public static void choose(String username) { GrantAccess admin = new GrantAccess(); GrantAccess user = new GrantUserAccess(); if (username.equals("admin")) { admin.displayAccountStatus(); } else { user.displayAccountStatus(); } } public static void main(String[] args) { choose("user"); } } |
...
In this compliant solution, the programmer declares the displayAccountStatus()
methods as instance methods, by removing the static
keyword. Consequently, the dynamic dispatch at the call sites produces the expected result. He also uses the The @Override
annotation to indicate indicates intentional overriding of the parent method.
Code Block | ||
---|---|---|
| ||
class GrantAccess {
public void displayAccountStatus() {
System.out.print("Account details for admin: XX");
}
}
class GrantUserAccess extends GrantAccess {
@Override
public void displayAccountStatus() {
System.out.print("Account details for user: XX");
}
}
public class StatMethod {
public static void choose(String username) {
GrantAccess admin = new GrantAccess();
GrantAccess user = new GrantUserAccess();
if (username.equals("admin")) {
admin.displayAccountStatus();
} else {
user.displayAccountStatus();
}
}
public static void main(String[] args) {
choose("user");
}
}
|
Wiki Markup |
---|
NoteYou thatcan "In a subclass, you can also overload the methods inherited from the superclass. Suchin overloadeda methodssubclass. neither hide nor override the superclass methodsâthey Overloaded methods are new methods, unique to the subclass." and neither hide nor override the superclass method \[[Tutorials 2008|AA. Bibliography#Tutorials 08]\]. |
Wiki Markup |
---|
Technically, a {{private}} method cannot be hidden or overridden. There is no requirement that {{private}} methods with the same signature in the subclass and the superclass, bear any relationship in terms of having the same return type or {{throws}} clause, the necessary conditions for _hiding_ \[[JLS 2005|AA. Bibliography#JLS 05]\]. Consequently, therehiding may be no _hiding_cannot occur when the methods have different return types or {{throws}} clauses. |
Exceptions
MET11-EX1EX0: Method hiding may occasionally be unavoidable. Declaration of hiding methods is permissible in such cases, provided that all invocations of hiding or hidden methods use qualified names or method invocation expressions that explicitly indicate which specific method will be is invoked. If the example above had been such a case, modification of the choose()
method as shown below would have been an acceptable alternative.
Code Block | ||
---|---|---|
| ||
public static void choose(String username) {
if (username.equals("admin")) {
GrantAccess.displayAccountStatus();
} else {
GrantUserAccess.displayAccountStatus();
}
}
|
...
Automated detection of violations of this guideline appear to be are straightforward. Automated determination of cases where method hiding was unavoidable appears to be infeasible. However, determining whether all invocations of hiding or hidden methods explicitly indicate which specific method will be invoked appears to be is invoked is straightforward.
Related Vulnerabilities
...