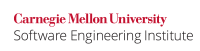
Wiki Markup |
---|
Programs must not catch {{java.lang.NullPointerException}}. A {{NullPointerException}} exception thrown at runtime indicates the existence of an underlying {{null}} pointer dereference that must be fixed in the application code (see rule [EXP01-J. Never dereference null pointers] for more information). Handling the underlying null pointer dereference by catching the {{NullPointerException}} rather than fixing the underlying problem is inappropriate for several reasons. First, catching {{NullPointerException}} adds significantly more performance overhead than simply adding the necessary null checks \[[Bloch 2008|AA. Bibliography#Bloch 08]\]. Second, when multiple expressions in a {{try}} block are capable of throwing a {{NullPointerException}}, it is difficult or impossible to determine which expression is responsible for the exception because the {{NullPointerException}} {{catch}} block handles any {{NullPointerException}} thrown from any location in the {{try}} block. Third, programs rarely remain in an expected and usable state after a {{NullPointerException}} has been thrown. Attempts to continue execution after first catching and logging (or worse, suppressing) the exception rarely succeed. |
Likewise, programs must not catch RuntimeException
or its ancestors, Exception
, or Throwable
. Few, if any, methods are capable of handling all possible runtime exceptions. When a method catches RuntimeException
, it may receive exceptions unanticipated by the designer, including NullPointerException
and ArrayIndexOutOfBoundsException
. Many catch
clauses simply log or ignore the enclosed exceptional condition and attempt to resume normal execution; this practice often violates rule ERR00-J. Do not suppress or ignore checked exceptions. Runtime exceptions often indicate bugs in the program that should be fixed by the developer and often cause control flow vulnerabilities.
...
This design choice suppresses genuine occurrences of NullPointerException
in violation of rule ERR00-J. Do not suppress or ignore checked exceptions. It also violates the design principle that exceptions should be used only for exceptional conditions ; because ignoring a null Log
object is part of the ordinary operation of a server.
...
An acceptable alternative implementation uses a setter method and a getter method accessor methods to control all interaction with the reference to the current log. The setter accessor method to set a log ensures use of the null object in place of a null reference. The getter accessor method to get a log ensures that any retrieved instance is either an actual logger or a null object (but never a null reference). Instances of the null object are immutable and are inherently thread-safe.
...
Noncompliant Code Example (Division)
In this This noncompliant code example , assumes that the original version of the division()
method is was declared to throw only ArithmeticException
. However, the caller catches the more general Exception
type to report arithmetic problems rather than catching the specific exception ArithmeticException
type. This practice is insecure risky because future changes to the method signature could add more exceptions to the list of potential exceptions the caller must handle. In this example, a newer version revision of the division()
method can potentially throw IOException
in addition to ArithmeticException
. However, the compiler cannot inform the caller's developer to provide will not diagnose the lack of a corresponding handler because his or her existing code the invoking method already catches IOException
as a result of catching Exception
. Consequently, the recovery process might be inappropriate for the specific exception type that is thrown. Furthermore, the developer has failed to anticipate that catching Exception
also catches unchecked exceptions.
Code Block | ||
---|---|---|
| ||
public class DivideException { public static void main(String[] args) { trydivision(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average division(200, 5); division(200, 0); = totalSum / totalNumber; // DivideAdditional byoperations zero that may } catch (Exception e) { throw IOException... System.out.println("DivideAverage: by" zero exception : " + average); } public static void main(String[] args) { try { division(200, 5); + e.getMessage());division(200, 0); // Divide by zero } catch } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { (Exception e) { System.out.println("Divide by zero exception : " int average = totalSum / totalNumber; // Additional operations that may throw IOException... + Systeme.out.println("Average: " + average);getMessage()); } } } |
Noncompliant Code Example
This noncompliant code example attempts improvement to solve the problem by specifically catching ArithmeticException
. However, it continues to catch Exception
and consequently catches both unanticipated checked exceptions and unanticipated runtime exceptions.
...
Code Block | ||
---|---|---|
| ||
import java.io.IOException; public class DivideException { public static void main(String[] args) { try { division(200, 5); division(200, 0); // Divide by zero } catch (ArithmeticException | IOException ex) { ExceptionReporter.report(ex); } } public static void division(int totalSum, int totalNumber) throws ArithmeticException, IOException { int average = totalSum / totalNumber; // Additional operations that may throw IOException... System.out.println("Average: "+ average); } } |
...
ERR08-EX0: A catch
block may catch all exceptions to process them before rethrowing them (filtering sensitive information from exceptions before the call stack leaves a trust boundary, for example). Refer to ruleERR01-J. Do not allow exceptions to expose sensitive information and weaknesses CWE 7 and CWE 388 for more information. In such cases, a catch
block should catch Throwable
rather than Exception
or RuntimeException
.
...
Wiki Markup |
---|
*ERR08-EX1:* Task processing threads such as worker threads in a thread pool or the Swing event dispatch thread are permitted to catch {{RuntimeException}} when they call untrusted code through an abstraction such as the {{Runnable}} interface \[[Goetz 2006|AA. Bibliography#Goetz 06], p. 161\]. |
...
Catching NullPointerException
may mask an underlying null
dereference, degrade application performance, and result in code that is hard to understand and maintain. Likewise, catching RuntimeException
, Exception
, or Throwable
may unintentionally trap other exception types and prevent them from being handled properly.
...