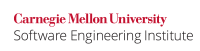
Programs must not use instance locks to protect static shared data because instance locks are ineffective when two or more instances of the class are created. Consequently, failure to use a static lock object leaves the shared state unprotected against concurrent access. Lock objects for classes that can interact with untrusted code must also be private and final, as shown in rule LCK00-J. Use private final lock objects to synchronize classes that may interact with untrusted code.
Noncompliant Code Example (
...
Nonstatic Lock Object for Static Data)
This noncompliant code example attempts to guard access to a the static counter
field using a non-static lock object. When two Runnable
tasks are started, they will create two instances of the lock object and will lock on each instance separately.
Code Block | ||
---|---|---|
| ||
public final class CountBoxes implements Runnable { private static volatile int counter; // ... private final Object lock = new Object(); @Override public void run() { synchronized (lock) { counter++; // ... } } public static void main(String[] args) { for (int i = 0; i < 2; i++) { new Thread(new CountBoxes()).start(); } } } |
...
This compliant solution ensures the atomicity of the increment operation by declaring the lock object as staticlocking on a static object.
Code Block | ||
---|---|---|
| ||
public class CountBoxes implements Runnable { private static int counter; // ... private static final Object lock = new Object(); public void run() { synchronized (lock) { counter++; // ... } } // ... } |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
LCK06-J | medium | probable | medium | P8 | L2 |
Automated Detection
The following table summarizes the examples flagged as violations by SureLogic Flashlight:
Noncompliant Code Example | Flagged | Message |
---|---|---|
non-static lock object for | No | No obvious issues |
method synchronization for | No | No obvious issues |
The following table summarizes the examples flagged as violations by SureLogic JSure:
...
Noncompliant Code Example
...
Flagged
...
Message
...
non-static lock object for static
data
...
Yes
...
modeling problem: static region "counter" should be protected by a static field
...
method synchronization for static
data
...
Yes
...
Some static analysis tools can detect violations of this rule.
Related Guidelines
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="071beb7ae5c2a80a-6172515d-43774e19-b6fdb421-e7d7fa22a3acda29ff77c648"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | ]]></ac:plain-text-body></ac:structured-macro> |
...