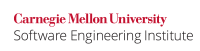
...
Wiki Markup |
---|
Task-specific recovery or clean-up actions can be performed by overriding the {{afterExecute()}} hook of the {{java.util.concurrent.ThreadPoolExecutor}} class. This hook is called either when a task concludes successfully by executing all statements in its {{run()}} method or when the task halts because of an exception. (Some implementations may fail to catch {{java.lang.Error}}. (See [Bug ID 6450211|http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=6450211] for more information \[[SDN 2008|AA. Bibliography#SDN 08]\].). When using this approach, substitute the executor service with a custom {{ThreadPoolExecutor}} that overrides the {{afterExecute()}} hook as shown below: |
Code Block | ||
---|---|---|
| ||
final class PoolService { // The values have been hard-coded for brevity ExecutorService pool = new CustomThreadPoolExecutor( 10, 10, 10, TimeUnit.SECONDS, new ArrayBlockingQueue<Runnable>(10)); // ... } class CustomThreadPoolExecutor extends ThreadPoolExecutor { // ... Constructor ... public CustomThreadPoolExecutor( int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue) { super(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue); } @Override public void afterExecute(Runnable r, Throwable t) { super.afterExecute(r, t); if (t != null) { // Exception occurred, forward to handler } // ... Perform task-specific clean-up actions } @Override public void terminated() { super.terminated(); // ... Perform final clean-up actions } } |
...
Code Block | ||
---|---|---|
| ||
final class PoolService { private static final ThreadFactory factory = new new ExceptionThreadFactory(new MyExceptionHandler()); private static final ExecutorService pool = Executors.newFixedThreadPool(10, factory); public void doSomething() { pool.execute(new Task()); // Task is a runnable class } public static class ExceptionThreadFactory implements ThreadFactory { private static final ThreadFactory defaultFactory = Executors.defaultThreadFactory(); private final Thread.UncaughtExceptionHandler handler; public ExceptionThreadFactory( Thread.UncaughtExceptionHandler handler) { this.handler = handler; } @Override public Thread newThread(Runnable run) { Thread thread = defaultFactory.newThread(run); thread.setUncaughtExceptionHandler(handler); return thread; } } public static class MyExceptionHandler extends ExceptionReporter implements Thread.UncaughtExceptionHandler { // ... @Override public void uncaughtException(Thread thread, Throwable t) { // Recovery or logging code } } } |
Wiki Markup |
---|
The {{ExecutorService.submit()}} method can be used (in place of the {{execute()}} method) to submit a task to a thread pool and obtain a {{Future}} object. When the task is submitted via {{ExecutorService.submit()}}, thrown exceptions will never reach the uncaught exception handler, because the thrown exception is considered to be part of the return status and is consequently wrapped in an {{ExecutionException}} and re-thrownrethrown by {{Future.get()}} \[[Goetz 2006|AA. Bibliography#Goetz 06]\]. |
...
This compliant solution invokes the ExecutorService.submit()
method to submit the task so that a Future
object can be obtained. It uses the Future
object to let the task re-throw rethrow the exception so that it can be handled locally.
...
Failure to provide a mechanism for reporting that tasks in a thread pool failed as a result of an exceptional condition can make it harder or impossible to find the source of the issue.
...
Related Guidelines
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a054baf69bdc4ee7-2129724a-44c2455c-af969860-47783f276ec29d8b81b7bed0"><ac:plain-text-body><![CDATA[ | [[API 2006 | AA. Bibliography#API 06]] | interfaces Interfaces | ]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="05c9dc00543724a8-716f0ec9-441e4b21-904fb6dd-688fcf2e1e21309ed2908043"><ac:plain-text-body><![CDATA[ | [[Goetz 2006 | AA. Bibliography#Goetz 06]] | Chapter 7.3: Handling abnormal thread termination , Handling Abnormal Thread Termination | ]]></ac:plain-text-body></ac:structured-macro> |
...